How to Convert Set to List in Python
-
Convert Set to List in Python Using the
list()
Method - Convert Set to List in Python Using List Comprehension
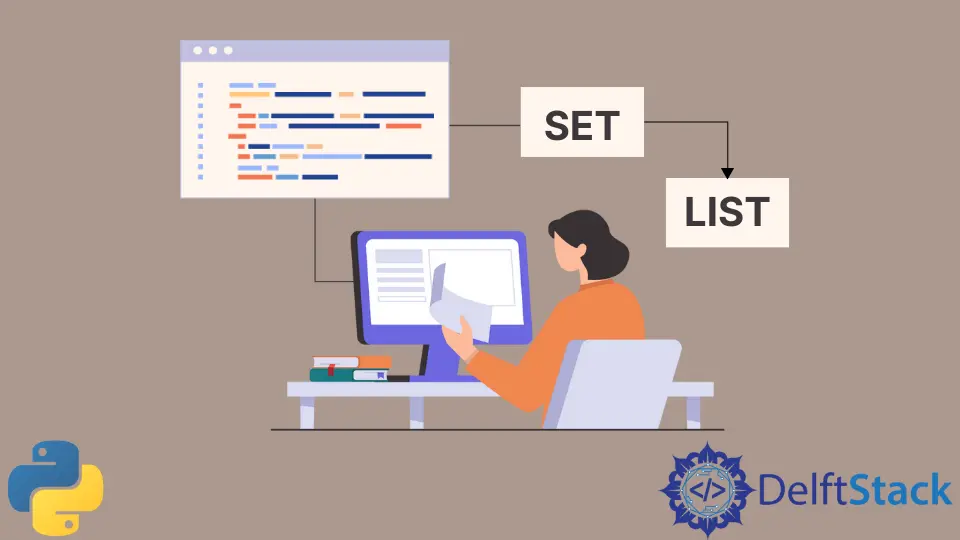
This tutorial will explain various methods of converting a set to a list in Python. The list is a standard data type in Python that stores values in sequence, and the set is also a standard data type in Python that stores values as an unordered collection.
The difference between a set and a list is that a set does not allow multiple occurrences of the same element and does not maintain the order of elements like a list does. As they have different properties, which means they have different built-in methods.
The various ways that can convert a set to a list are explained below.
Convert Set to List in Python Using the list()
Method
The list()
method in Python takes an iterable object as input and returns a list as output. If no parameter is passed to the method, it returns an empty list.
As a set is also an iterable object, we can pass it to the list()
method and convert it to a list. The code example below demonstrates how to use the list()
method to convert a set to a list in Python.
myset = set(["Amsterdam", "Paris", "London", "New York", "Tokyo"])
mylist = list(myset)
print(type(mylist))
Output:
<class 'list'>
Convert Set to List in Python Using List Comprehension
List comprehension is a comprehensive syntactic way of creating a new list from an iterable object. We can use list comprehension to perform some action or filter on the iterable object while creating a new list, like replacing some value or converting a string type to an integer, etc.
We can implement the list comprehension in Python using the following syntaxes.
- Simple list comprehension:
mylist = [f(x) for x in iterable]
- Conditional list comprehension:
mylist = [f(x) if condition else g(x) for x in iterable]
As we want to convert the whole set to a list, we will use the first syntax. The example code below demonstrates how to use list comprehension to convert a set to a list in Python:
myset = set(["Lahore", "Delhi", "London", "New York", "Tokyo"])
mylist = [x for x in myset]
print(type(mylist))
Output:
<class 'list'>
Related Article - Python Set
- How to Join Two Sets in Python
- How to Add Values to a Set in Python
- How to Check if a Set Is a Subset of Another Set in Python
- How to Convert Set to String in Python
- Python Set Pop() Method
- How to Create a Set of Sets in Python
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python