How to Set HTTP Proxy Settings in Python
-
Use
export
to Set HTTP Proxy Settings in Python -
Use
set
to Set HTTP Proxy Settings in Python -
Use
--proxy
to Set HTTP Proxy Settings in Python -
Use
proxies
to Set HTTP Proxy Setting in Python
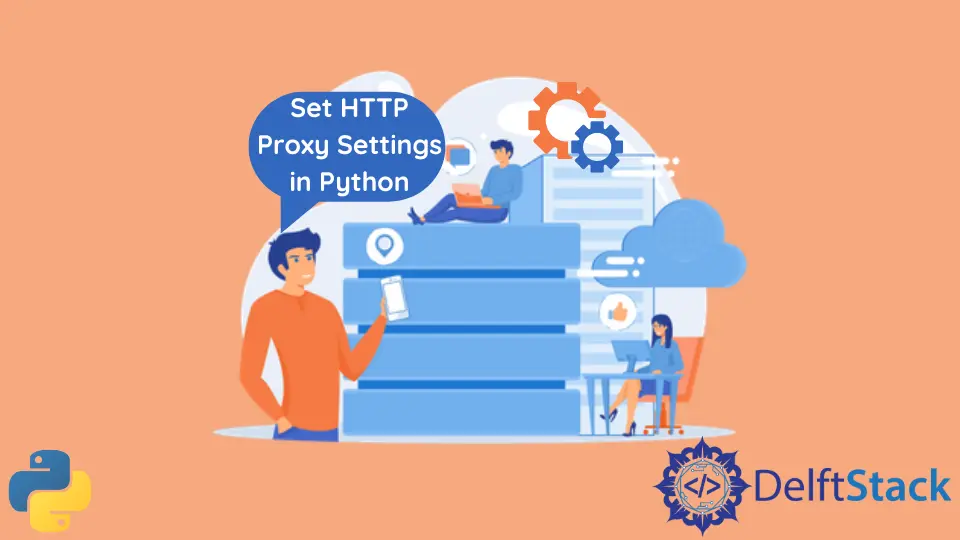
Working within Python, we might connect to external servers from typical network requests to pip
package installations. If you are behind the HTTP proxy, you might experience a connection timeout.
To solve this issue, you need to configure your HTTP proxy setting, and more natively, this is an operating system (OS) issue, and some commands can suffice.
However, we can also use specific parameters within a third-party library to specify the proxies we intend to use without changing our native OS proxy.
In this article, we will discuss our to set HTTP proxy settings on our PC and within Python.
Use export
to Set HTTP Proxy Settings in Python
As said earlier, configuring HTTP proxies is a more native operation and will defer based on the OS you are using.
For Linux and macOS, you can use the export
command and specify the http_proxy
with the HTTP proxy you intend to use.
export http_proxy="username:password@ip address:port number"
Also, you can use https_proxy
to update the HTTPS proxy.
export https_proxy="username:password@ip address:port number"
It is important to note that this HTTP proxy setting configuration is only for the terminal session you execute it for and is not global; and is most valuable, especially if the connection timeout is specific to only that Python operation.
To make it global, you need to add the above command to your ~/.bash_profile
for Linux or Older Macs or ~/.zshrc
for newer macOS.
Use set
to Set HTTP Proxy Settings in Python
To configure your HTTP and HTTPS proxy setting in Windows, you need the same variables as within Linux and macOS environments, http_proxy
and https_proxy
. However, the command to set the proxy settings is set
.
set http_proxy="username:password@ip address:port number"
And for the HTTPS proxy.
set https_proxy="username:password@ip address:port number"
As with the Linux and macOS environments, the HTTP and HTTPS configuration is only functional for the terminal session.
Use --proxy
to Set HTTP Proxy Settings in Python
If you are experiencing connection timeout or HTTP issues when working or downloading Python packages, you can use the --proxy
argument.
pip install --proxy="username:password@ip address:port number" package-name
The above commands allow pip
to use a proxy server to access sites; if a password is required, it will ask for it.
Use proxies
to Set HTTP Proxy Setting in Python
When working with API or external websites, you can use proxies to bypass filters, increase your anonymity, and maintain your security.
To use such proxies, you can use the requests
package and create a dictionary holding the proxies for both HTTP and HTTPS.
proxies = {
"http": "http://211.161.103.139",
"https": "http://63.239.220.5",
}
To install the requests
package, use the pip
command given below:
pip install requests
To use the proxies
argument, we can pass the URL
and the proxies
.
import requests as req
proxies = {
"http": "http://211.161.103.139",
"https": "http://63.239.220.5",
}
URL = "https://jsonplaceholder.typicode.com/todos/1"
response = req.get(URL)
print(response)
If the proxies are working, the output of the code would be a status code (200) that indicates success. It means that the resource we are looking for at the URL has been fetched.
<Response [200]>
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn