How to Get Difference in Sets in Python
-
Find Difference in Sets in Python Using
set.symmetric_difference()
Method -
Find Difference in Sets in Python Using the
for
Loop -
Find Difference in Sets in Python Using the
XOR
Operator
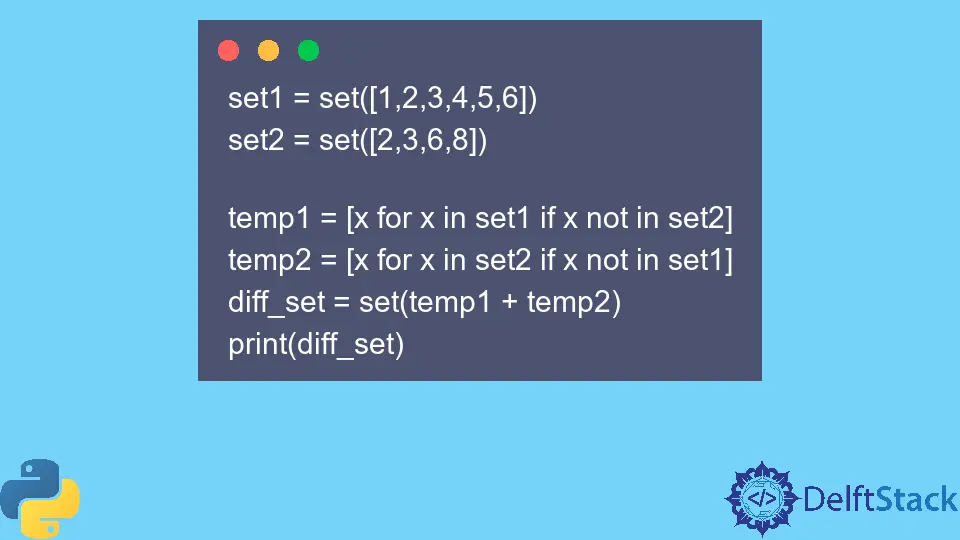
This tutorial will explain the various ways to find the difference between the two sets in Python. By the difference, we mean the elements which are not common between the two sets.
For example:
set1 = set([1, 2, 3, 4, 5, 6])
set2 = set([2, 3, 6, 8])
The difference between these two sets should be 1,4,5,8
.
Find Difference in Sets in Python Using set.symmetric_difference()
Method
The set.symmetric_difference()
method takes another set as input and returns the difference between them. The below example code explains how to use set.symmetric_difference()
to get the elements that are not common in both sets and get the required difference set.
set1 = set([1, 2, 3, 4, 5, 6])
set2 = set([2, 3, 6, 8])
diff_set = set1.symmetric_difference(set2)
print(diff_set)
Output:
{1, 4, 5, 8}
Find Difference in Sets in Python Using the for
Loop
To find the difference between the two sets, we can use the for
loop to iterate through both sets and check if there is the same element in the other set and add it to the list if they are unique. And the elements which exist in both sets will be ignored.
Example code:
set1 = set([1, 2, 3, 4, 5, 6])
set2 = set([2, 3, 6, 8])
temp1 = [x for x in set1 if x not in set2]
temp2 = [x for x in set2 if x not in set1]
diff_set = set(temp1 + temp2)
print(diff_set)
Output:
{8, 1, 4, 5}
Find Difference in Sets in Python Using the XOR
Operator
A simple way to find the difference between the sets is to apply the XOR
operation on them, and this will drop the same elements and will only return elements that are not the same in both sets as required.
To implement this in code, we can use the ^
operator between two sets to get the desired set difference.
set1 = set([1, 2, 3, 4, 5, 6])
set2 = set([2, 3, 6, 8])
diff_set = set(set1) ^ set(set2)
print(diff_set)
Output:
{1, 4, 5, 8}