How to Implicit Wait With Selenium in Python
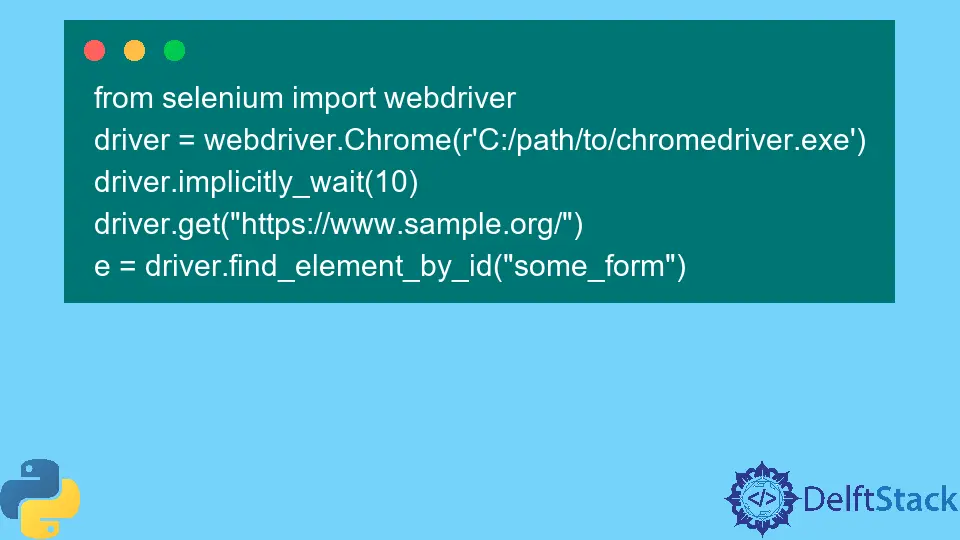
The selenium
package is used for automation and testing with Python scripts. We can use it to access individual elements from the webpage and work with them.
There are many methods available in this package to retrieve the elements based on different attributes. When a page is loaded, some elements are retrieved dynamically.
These elements may load at a different speed as compared to the rest.
Implicit Wait With Selenium in Python
If we try to get an element that is not available, an ElementNotVisibleException
is raised. This happens because the element is defined in the source but not yet visible in the DOM.
For this, we can use implicit waits. With implicit waits using selenium
, we can tell the webdriver
object to wait for the required time before throwing an exception.
The exception is thrown if the required element is not found within this period.
We use the implicitly_wait()
function to set the implicit wait time. This function is used with webdriver
to specify the implicit wait time.
The time is specified as seconds.
See the code below.
from selenium import webdriver
driver = webdriver.Chrome(r"C:/path/to/chromedriver.exe")
driver.implicitly_wait(10)
driver.get("https://www.sample.org/")
e = driver.find_element_by_id("some_form")
In the above example, we redirect to a web page using the webdriver
object and try to retrieve an element using the find_element_by_id()
function. This function will find the element whose id
attribute matches the value provided.
Since this is a dynamic element, we specify an implicit time of ten seconds using the implicitly_wait()
method to ensure that the element gets time to load.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Selenium
- How to Check if Element Exists Using Selenium Python
- How to Refresh Page in Python Selenium
- WebDriverException: Message: Geckodriver Executable Needs to Be in PATH Error in Python
- How to Install Python Selenium in macOS
- How to Login to a Website Using Selenium Python
- How to Open and Close Tabs in a Browser Using Selenium Python