How to Implement Selection Sort Algorithm in Python
- Iterative Method to Implement Selection Sort in Python
- Recursive Method to Implement Selection Sort in Python
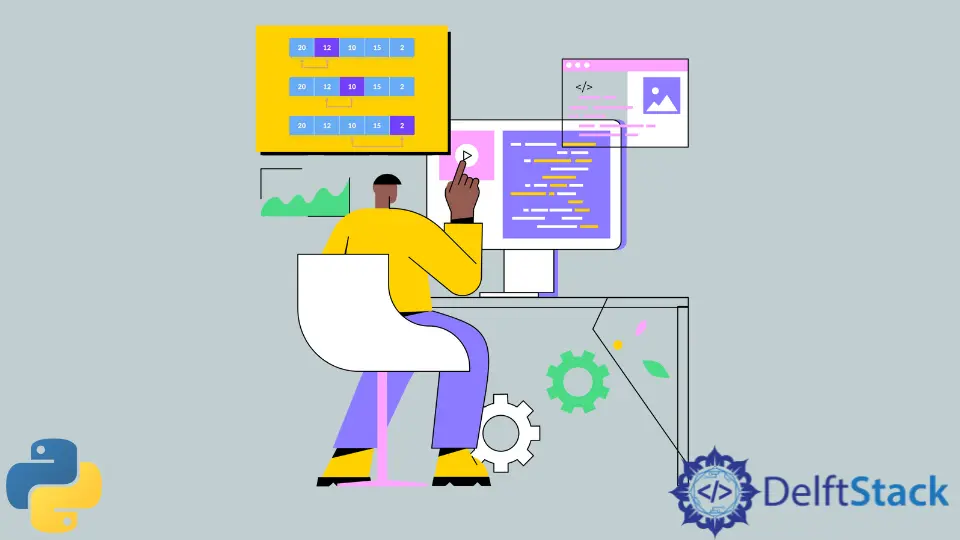
This article discusses the selection sort algorithm and how to implement it in Python.
Selection sort is one of the algorithms for sorting small-sized data structures. A basic comparison-based algorithm can divide the given array into two parts: the sorted
part (left) and the unsorted
part (right).
The algorithm aims to find the element with the minimum value from the unsorted
part and sends it to the sorted
part. This process is repeated until the unsorted
part of the given array contains no elements.
throughout the run of the selection sort algorithm, the given array is divided into two subarrays:
- One subarray contains all the sorted elements.
- Another subarray contains all the unsorted elements that are yet to be checked.
There are two approaches to implement the selection sort algorithm: iterative selection sort
and recursive selection sort
.
Iterative Method to Implement Selection Sort in Python
A simple iterative approach can be utilized to execute selection sort in Python.
This method takes one element with the smallest value from the unsorted sub-array and puts it into the sorted sub-array in every iteration of the for
loop.
Sample code using the iterative method to implement selection sort in Python.
def selectionSort(array, size):
for step in range(size):
min_a = step
for i in range(step + 1, size):
if array[i] < array[min_a]:
min_a = i
(array[step], array[min_a]) = (array[min_a], array[step])
X = [45, 22, 18, 32, 49]
size = len(X)
selectionSort(X, size)
print("Sorted Array is:")
print(X)
Output:
Sorted Array is:
[18,22,32,45,49]
Recursive Method to Implement Selection Sort in Python
The recursion process is another method to do selection sort in Python. We create a function that calls for the unsorted part recursively under itself.
Sample code that uses the recursive method to implement selection sort in Python.
def minIndex(a, x, y):
if x == y:
return x
z = minIndex(a, x + 1, y)
return x if a[x] < a[z] else z
def RSS(a, n, index=0):
if index == n:
return -1
z = minIndex(a, index, n - 1)
if z != index:
a[z], a[index] = a[index], a[z]
RSS(a, n, index + 1)
l1 = [5, 7, 2, 4, 6]
n = len(l1)
RSS(l1, n)
for x in l1:
print(x, end=" ")
Output:
2 4 5 6 7
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn