How to Solve Python Segmentation Fault 11
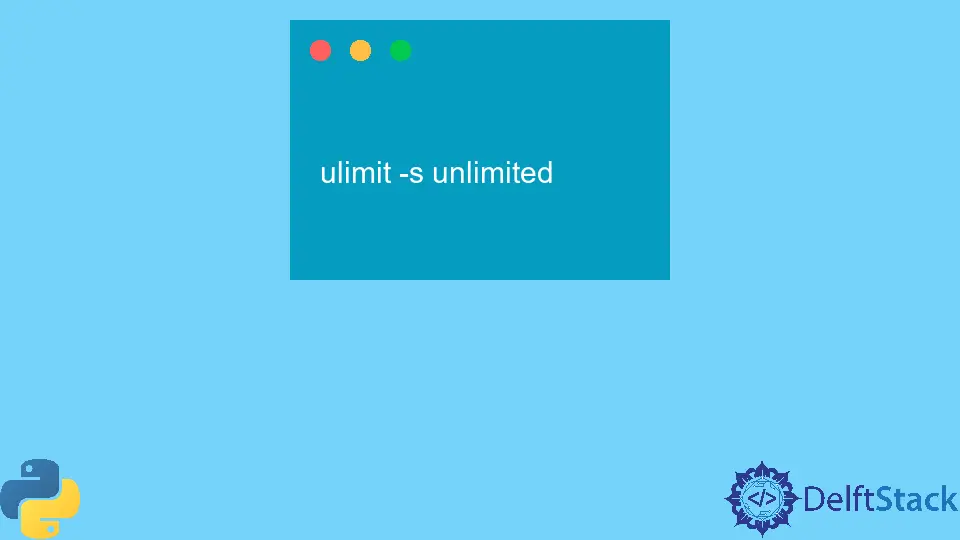
This tutorial will discuss possible ways to fix the Segmentation fault: 11
in Python.
Fix Segmentation fault: 11
in Python
Segmentation fault: 11
is a common error that occurs when a program in the terminal tries to access a memory location that it’s not supposed to. This error can occur while running Python in the terminal on OS X for various reasons, such as a bug in the code, a memory leak, or a problem with the system’s memory management.
Below are a few ways to troubleshoot and fix the Segmentation fault: 11
error while running Python in the terminal on OS X.
-
Check for bugs in the code: The first step is to check the code for any bugs that may be causing the error. Look for any potential memory leaks or issues with memory management.
-
Increase the stack size: Sometimes, increasing the stack size can help fix the
Segmentation fault: 11
error. You can do this by running the following command in the terminal.ulimit -s unlimited
-
Use a debugging tool: A debugging tool such as GDB (GNU Debugger) can help identify the source of the error.
-
Update the Python version: If the issue persists, you may want to try updating the Python version to see if that fixes the problem.
-
Re-install Python and all the packages: Sometimes, the issue could be with the package installation; in that case, try to remove Python and re-install it with all the packages needed.
-
Check the system memory: Make sure that the system has enough memory and that no other programs that consume a lot of memory are running.
It is important to note that theSegmentation fault: 11
error can be caused by various factors, and no single solution will work for everyone. The best approach is to try the above methods one by one until the issue is resolved.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python