Scientific Notation in Python
-
Use the
format()
Function to Represent Values in Scientific Notation in Python -
Use the
fstrings
to Represent Values in Scientific Notation in Python -
Use the
numpy.format_float_scientific()
Function to Represent Values in Scientific Notation
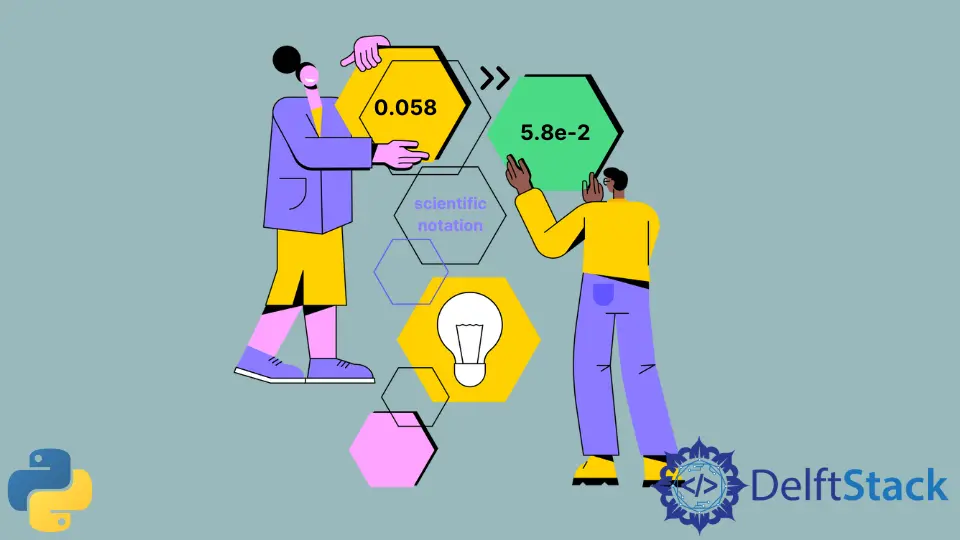
Scientific notation is used for representing very large or small numbers in a compact and understandable way. Such notation is also used in programming; for example, 6000000 can be represented as 6E+6
. Similarly, 0.000006
can be represented as 6E-6
.
In this tutorial, we will discuss how to use such scientific notations in Python.
Use the format()
Function to Represent Values in Scientific Notation in Python
The format
function is used for formatting strings in a specific format. We can use this function to convert large or small float values to their respective exponential format as shown below:
no1 = float(5800000.00000)
no2 = float(0.0000058)
print(format(no1, ".1E"))
print(format(no2, ".1E"))
Output:
5.8E+06
5.8E-06
Here .1E
is the specified formatting pattern. E
indicates exponential notation to print the value in scientific notation, and .1
specifies that there has to be one digit after the decimal.
Use the fstrings
to Represent Values in Scientific Notation in Python
In Python 3.6 and up, we can use fstrings
to format strings. This method is considered to be more precise and less prone to errors. It improves the readability of the code compared to the traditional format()
function. fstrings
can help in representing, as shown below.
no1 = float(5800000.00000)
no2 = float(0.0000058)
print(f"{no1:.1E}")
print(f"{no2:.1E}")
Output:
5.8E+06
5.8E-06
Notice that the format pattern is the same as the above method.
Use the numpy.format_float_scientific()
Function to Represent Values in Scientific Notation
The NumPy
module in Python has a function format_float_scientific()
that can be used to format a floating value to its scientific notation.
We can use the precision
parameter to specify the total decimal digits and the exp_digits
to tell how many digits are needed in the exponential notation. Other parameters available are sign
, unique
, and trim
, which offer more customizations.
The final result returned from this function is a string.
import numpy as np
no1 = float(5800000.00000)
no2 = float(0.0000058)
print(np.format_float_scientific(no1, precision=1, exp_digits=3))
print(np.format_float_scientific(no2, precision=2, exp_digits=3))
Output:
5.8e+006
5.8e-006
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn