How to Run Python File From Python Shell
-
Use the
exec
Function to Run a Python File From Python Shell -
Use the
$ python
Keyword to Execute a Python File From Python Shell
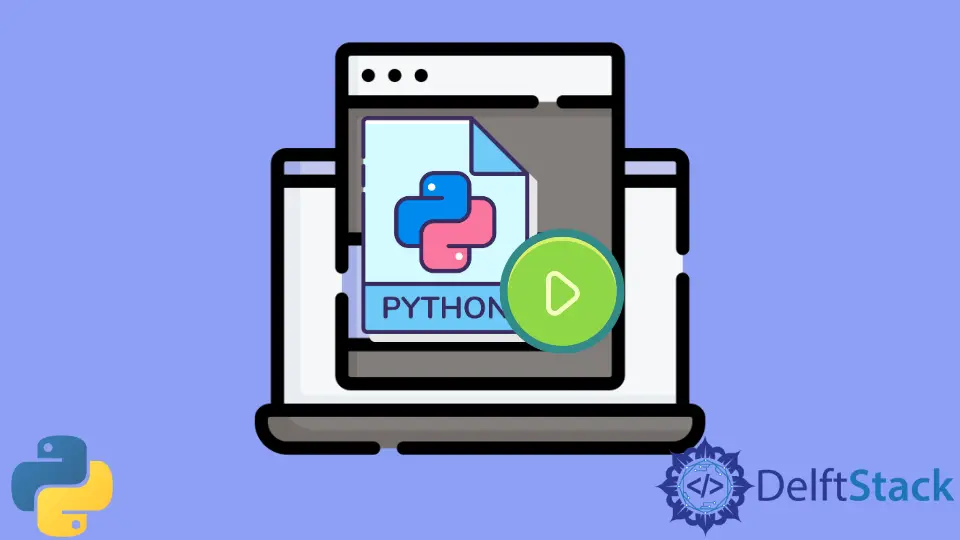
Python is an interpreter language, which means that it executes the code line by line. It also provides a Python Shell, which executes a single Python command and then displays the result.
It is also popularly known as R(read) E(evaluate)) P(print) L(loop)
- REPL
, where it reads the command and then evaluates the command and ultimately prints the result, and loops it back to the start to read the command again.
Use the exec
Function to Run a Python File From Python Shell
The exec()
function helps in dynamically executing the code of a python program. We can pass the code as a string or an object code.
It executes the object code as it is while the string is checked for syntactical errors, if any. If there is no syntactical error, then the parsed string is executed as a Python statement.
For example in Python3,
exec(open("C:\\any_file_name.py").read())
For example in Python2,
execfile('C:\\any_file_name.py')
Use the $ python
Keyword to Execute a Python File From Python Shell
$ python
can be used in the command prompt to trigger it to run a Python file. However, for $ python
to work seamlessly, the project program should follow the structure:
# Suppose this is the file you want to run from Python Shell
def main():
"""core of the program"""
print("main fn running")
if __name__ == "__main__":
main()
Following this structure, we can use the $ python
in the command prompt as follows :
$ python any_file_name.py
If you want to run the main function, do use the following command:
import _any_file_name
_any_file_name.main() #this command calls the main function of your program.