How to Run Bash Scripts in Python
- Method 1: Using the subprocess Module
- Method 2: Using os.system
- Method 3: Using subprocess.Popen
- Conclusion
- FAQ
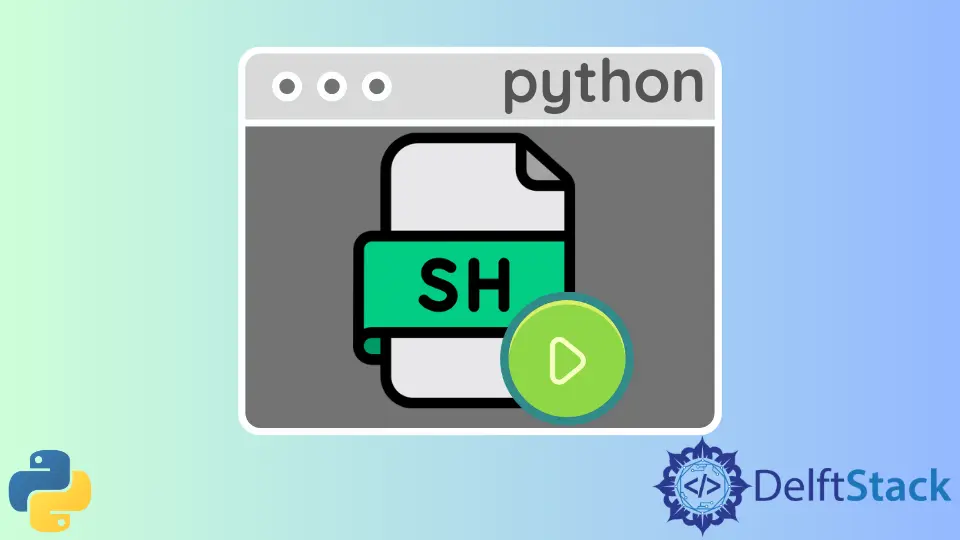
Executing Bash scripts from Python can significantly enhance your automation capabilities and streamline your workflows. Whether you are a developer looking to integrate system commands into your applications or a data scientist wanting to leverage shell capabilities, understanding how to execute Bash scripts using Python is essential.
In this article, we will explore various methods for executing Bash scripts through Python, including the use of the subprocess module, os.system, and more. By the end of this guide, you will have a solid understanding of how to seamlessly run Bash commands and scripts within your Python projects, allowing you to harness the full power of both languages.
Method 1: Using the subprocess Module
The subprocess
module is a powerful tool in Python that allows you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. This method is preferred for executing Bash scripts because it provides more control and flexibility than other methods.
Here is a simple example of how to use the subprocess
module to execute a Bash script:
import subprocess
# Define the command to execute
command = ["bash", "your_script.sh"]
# Execute the command
process = subprocess.run(command, capture_output=True, text=True)
# Print the output and error (if any)
print("Output:")
print(process.stdout)
print("Error:")
print(process.stderr)
Output:
example of output
The subprocess.run
function executes the specified command and waits for it to complete. The capture_output=True
argument allows you to capture the output and error messages, which are then accessible through the stdout
and stderr
attributes of the returned process object. This method is advantageous because it allows you to handle errors gracefully by examining the stderr
output. Additionally, using lists to define commands helps avoid shell injection vulnerabilities.
Method 2: Using os.system
The os.system
function is another straightforward way to execute Bash scripts from Python. While it is simpler to use than subprocess
, it is less flexible and does not provide direct access to the output or error messages.
Here’s how you can use os.system
to run a Bash script:
import os
# Define the command to execute
command = "bash your_script.sh"
# Execute the command
exit_code = os.system(command)
# Print the exit code
print("Exit Code:", exit_code)
Output:
example of output
The os.system
function executes the command in a subshell. It returns the exit code of the command, which you can use to determine whether the script ran successfully. However, one limitation of this method is that you cannot directly capture the output of the executed script, which may be a drawback for more complex tasks. If you need to handle output or errors, consider using subprocess
instead.
Method 3: Using subprocess.Popen
For more advanced use cases, the subprocess.Popen
class provides even greater control over the execution of Bash scripts. This method allows you to interact with the process while it is running, making it suitable for long-running scripts or those that require real-time input.
Here’s an example of executing a Bash script using subprocess.Popen
:
import subprocess
# Define the command to execute
command = ["bash", "your_script.sh"]
# Open the process
process = subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
# Read output and error
stdout, stderr = process.communicate()
# Print the output and error
print("Output:")
print(stdout)
print("Error:")
print(stderr)
Output:
example of output
In this method, subprocess.Popen
starts the process and allows you to interact with it using pipes. The communicate()
method reads the output and error messages after the process has completed. This approach is particularly useful for long-running scripts, as it allows you to handle output in real-time. Additionally, you can provide input to the script if necessary, making this method highly versatile.
Conclusion
In conclusion, executing Bash scripts using Python can significantly enhance your programming capabilities, allowing for greater automation and efficiency. The three methods discussed—using the subprocess
module, os.system
, and subprocess.Popen
—each have their unique advantages and use cases. Depending on your needs, you can choose the most suitable method for your project. By mastering these techniques, you can seamlessly integrate Bash scripts into your Python applications, unlocking new possibilities for automation and system management.
FAQ
-
What is the best method to execute a Bash script from Python?
The best method depends on your specific needs. For most cases, using thesubprocess
module is recommended due to its flexibility and error handling capabilities. -
Can I capture the output of a Bash script in Python?
Yes, you can capture the output using thesubprocess
module by settingcapture_output=True
or usingsubprocess.PIPE
withPopen
. -
Is it safe to execute Bash scripts from Python?
Yes, but be cautious of shell injection vulnerabilities. When usingsubprocess
, it’s safer to pass commands as a list rather than a single string. -
Can I pass arguments to my Bash script from Python?
Yes, you can pass arguments by including them in the command list when usingsubprocess
oros.system
. -
What if my Bash script requires user input?
You can usesubprocess.Popen
to interact with the process and provide input as needed.