How to Reverse Order Using Slicing in Python
Shivam Arora
Feb 02, 2024
Python
Python Slicing
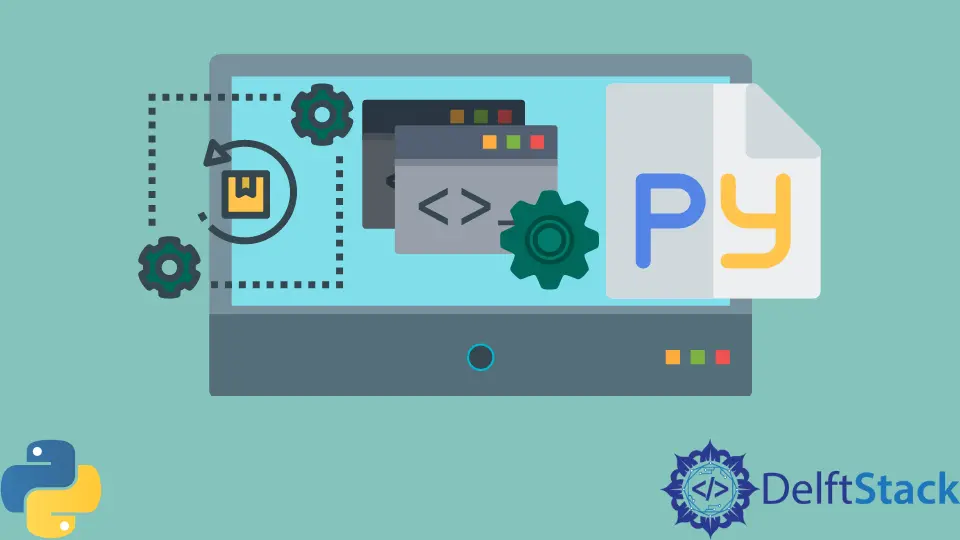
This article will demonstrate slicing in Python and the meaning of object[::-1]
in slicing. Here object represents an iterable that can be sliced like a string or a list.
Slicing allows the user to access parts of a sequence like lists, tuples, and strings. Many functions can be performed using slicing, for instance, deleting items or modify items in a sequence.
First, let us understand the syntax for slicing.
a[start: stop]
The items start from the given point and slices till stop -1.a[start: ]
The items start from the given point and slice till the end of an array.a[: stop]
The items start from the beginning and slice till stop -1.a[: ]
This gives the whole array as output.a[start: stop: step]
The items start from the given point and slices till stop -1 with provided step size.
The code below demonstrates the slicing in Python.
a = [1, 3, 5, 7, 9]
print(a[-1])
print(a[-2:])
print(a[:-2])
Output:
9
[7, 9]
[1, 3, 5]
Using a[::-1]
in Python to Reverse an Object Like an Array or String
As we saw above, we have a[start: stop: step]
step in slicing, and -1 means the last element of the array. Therefore, a[::-1]
starts from the end till the beginning reversing the given sequence that was stored.
For example,
a = "12345"
print(a[::-1])
Output:
54321
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe