How to Resize Image in Python
- Use the opencv module to resize images in Python
- Use the scikit-image module to resize images in Python
- Create a user-defined function to resize images in Python
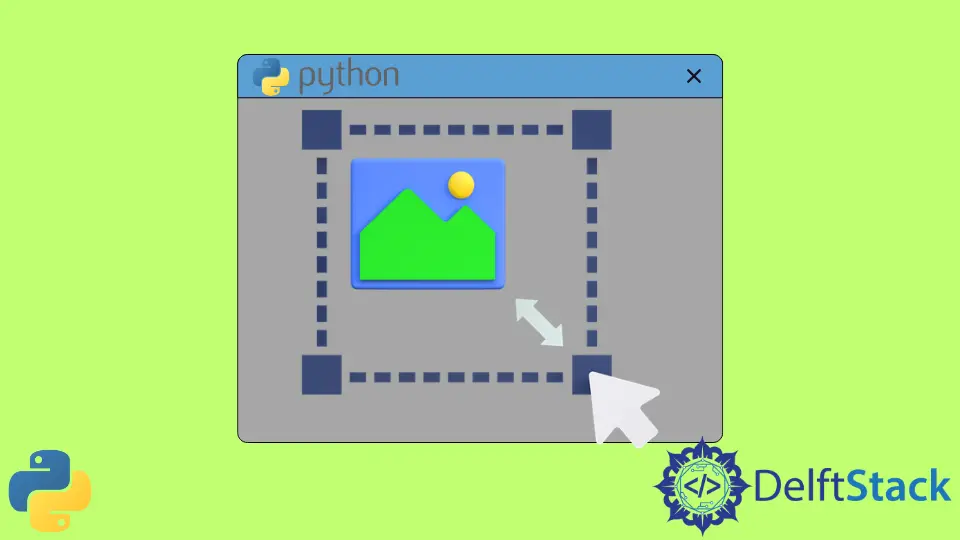
In this tutorial, we will discuss how to resize an image.
Essentially, we will resize the size of the numpy array, which represents an image. There is no direct functionality in the numpy module to achieve this. We cannot directly use the resize()
function because it disregards the axis and does not apply interpolation or extrapolation.
Note that after resizing, we can export this resized array and save it as an image. This is common for all the methods discussed below
Use the opencv module to resize images in Python
The OpenCV module is widely used in Python for image processing and computer vision. To resize an image, we will first read the image using the imread()
function and resize it using the resize()
function as shown below.
import cv2
import numpy as np
img = cv2.imread("filename.jpeg")
res = cv2.resize(img, dsize=(54, 140), interpolation=cv2.INTER_CUBIC)
The imread()
returns an array that stores the image. We resize it with the resize()
function. An important aspect here is the interpolation
parameter, which essentially tells how to resize an image. There are several ways to resize the image like INTER_NEAREST
, INTER_LINEAR
, and more. There is no best way to select this parameter; it differs from situation to situation.
Use the scikit-image module to resize images in Python
This module is built on the numpy library and has the resize()
function, which can effectively resize images. It can work on a variety of channels while taking care of interpolation, anti-aliasing, etc.
The following code shows how to use this function.
from skimage.transform import resize
import matplotlib.pyplot as plt
im = plt.imread("filename.jpeg")
res = resize(im, (140, 54))
Note that we use the matplotlib.pyplot.imread()
function to read the image in the above method. It can be substituted with any method of your preference.
Create a user-defined function to resize images in Python
We can also create our own function to achieve resizing in Python. It should be noted that this method is a basic resizing function, independent of any libraries, and will not perform interpolation, anti-aliasing as the above methods will.
The following code demonstrates this function.
import matplotlib.pyplot as plt
def scale(im, nR, nC):
number_rows = len(im) # source number of rows
number_columns = len(im[0]) # source number of columns
return [
[im[int(number_rows * r / nR)][int(number_columns * c / nC)] for c in range(nC)]
for r in range(nR)
]
im = plt.imread("filename.jpeg")
res = scale(im, 30, 30)
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn