Requests Headers in Python
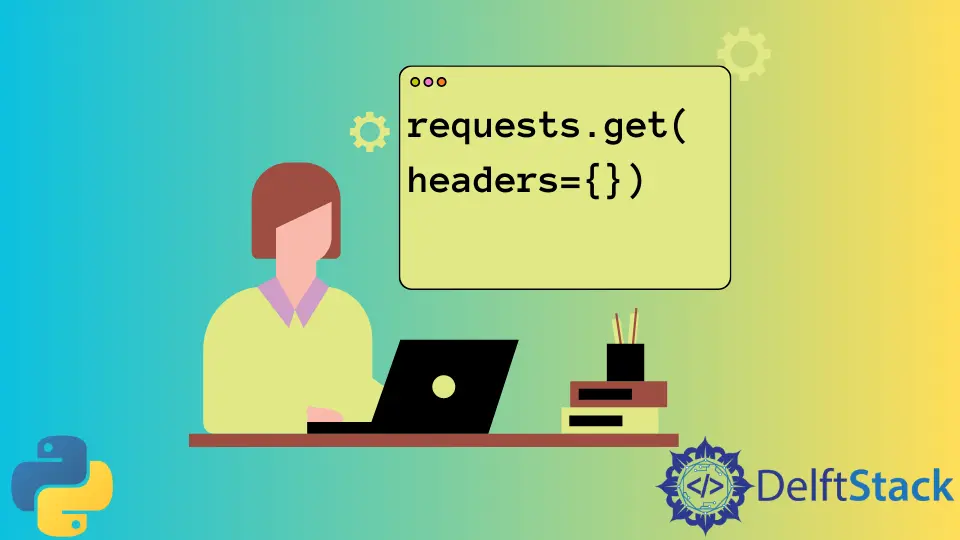
The requests
library can be defined as an efficient library utilizing HTTP requests in Python. This tutorial discusses the requests library and how to implement its functions in Python.
Requests Headers in Python
The functions within the requests
library make sending HTTP/1.1
requests easy in Python.
The need to manually add query strings to the URLs has been eliminated with the help of this library. Moreover, there is an automatic HTTP connection pooling and keep-alive.
Although many functions are available to help get a request in Python, we can utilize the requests.get()
function to implement python request headers. The syntax for this function is given for ease of understanding.
requests.get(url, params=None, headers=None, cookies=None, auth=None, timeout=None)
The requests.get()
functions returns a Response
object as the output.
The requests.get()
function contains several parameters, some of which are mandatory while the others are optional. All of these parameters have been explained below.
url
: Takes in the URL for the newly createdRequest
object/params
: An optional argument refers to theGET
parameters in a Python Dictionary that is to be sent along with theRequest
.headers
: An optional argument marks the HHTP Requests in a Python Dictionary that is to be sent along with theRequest
.cookies
: An optional argument marks theCookieJar
object to be sent along with theRequest
.auth
: Another optional argument, which marks theAuthObject
that is utilized to enable basictimeout
: Another optional parameter is a floating-point number utilized to describe the mentioned request’s timeout.
In this particular article, we will describe how to get the headers with the help of the requests
library.
Example:
import requests
r = requests.get("http://www.delftstack.com/", headers={"Content-Type": "text"})
print(r.headers["Content-Type"])
The above code provides the following Output:
text/html; charset=UTF-8
The above code can store all the headers with the requests.get()
function.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn