How to Remove \n From the String in Python
-
Remove
\n
From the String in Python Using thestr.strip()
Method -
Remove
\n
From String Usingstr.replace()
Method in Python -
Remove
\n
From String Usingregex
Method in Python
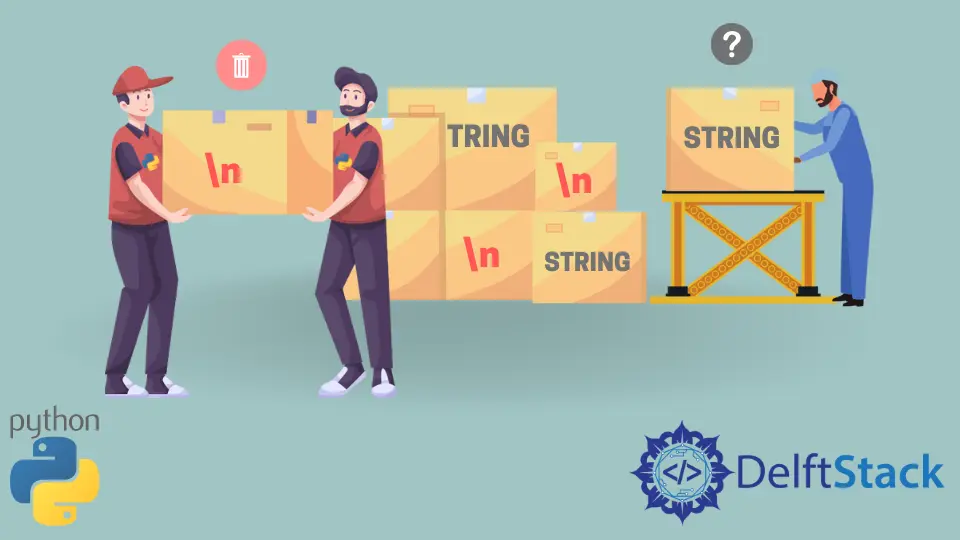
In this tutorial, we will look into the different ways to remove \n
and \t
from a string.
Remove \n
From the String in Python Using the str.strip()
Method
In order to remove \n
from the string using the str.strip()
method, we need to pass \n
and \t
to the method, and it will return the copy of the original string after removing \n
and \t
from the string.
str.strip()
method only removes the substrings from the string’s start and end position.Example code:
string = "\tHello, how are you\n"
print("Old String:")
print("'" + string + "'")
string = string.strip("\n")
string = string.strip("\t")
print("New String:")
print("'" + string + "'")
Output:
Old String:
' Hello, how are you?
'
New String:
'Hello, how are you?'
Remove \n
From String Using str.replace()
Method in Python
The other way to remove \n
and \t
from a string is to use the str.replace()
method. We should keep in mind that the str.replace()
method will replace the given string from the whole thing, not just from the string’s start or end. If you only need to remove something from the start and end only, you should use the str.strip()
method.
The str.replace()
method two arguments as input, first is the character or string you want to be replaced, and second is the character or string you want to replace with. In the below example, since we just wanted to remove \n
and \t
, we have passed the empty string as the second argument.
Example code:
string = "Hello, \nhow are you\t?\n"
print("Old String:")
print("'" + string + "'")
string = string.replace("\n", "")
string = string.replace("\t", "")
print("New String:")
print("'" + string + "'")
Output:
Old String:
'Hello,
how are you ?
'
New String:
'Hello, how are you?'
Remove \n
From String Using regex
Method in Python
To remove \n
from the string, we can use the re.sub()
method. The below code example demonstrates how to remove \n
using the re.sub()
method. \n
is the new line’s regular express pattern, and it will be replaced with the empty string - ""
.
import re
string = "Hello, \nhow are you\n?"
print("Old String:")
print("'" + string + "'")
new_string = re.sub(r"\n", "", string)
print("New String:")
print("'" + new_string + "'")
Output:
Old String:
'Hello,
how are you
?'
New String:
'Hello, how are you?'