How to Remove List Element by Index in Python
-
Remove List Element by Index With
del
Keyword in Python -
Remove List Element by Index With
pop()
Function in Python
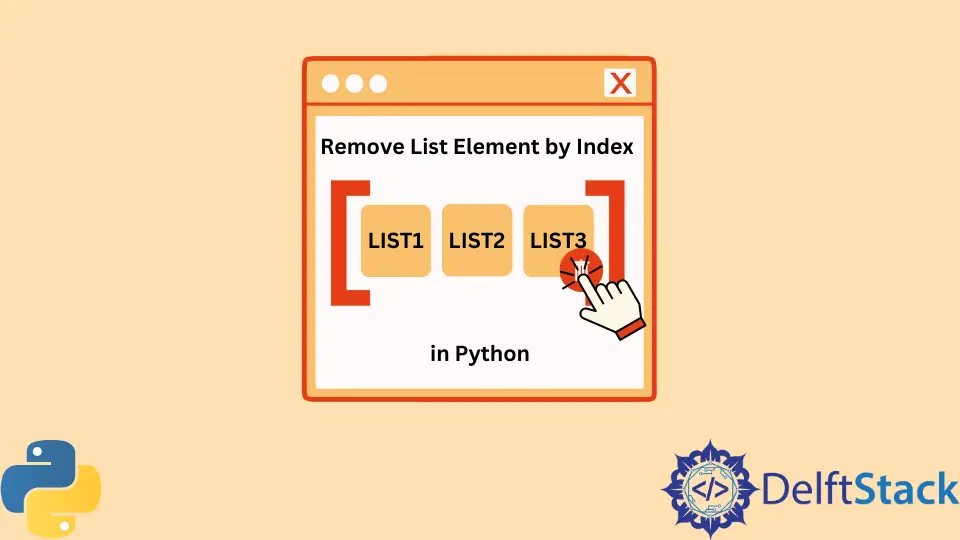
In this tutorial, we will discuss methods to remove list elements by index in Python.
Remove List Element by Index With del
Keyword in Python
The del
statement is used to delete objects in Python. The del
statement can also be used to remove list elements by index. The following code example shows us how we can remove list elements by index with the del
keyword in Python.
list1 = [0, 1, 2, 3, 4]
del list1[1]
print(list1)
Output:
[0, 2, 3, 4]
In the above code, we first initialize a list and then remove the element at index 1
of the list using the del
keyword.
Remove List Element by Index With pop()
Function in Python
The pop()
function is used to remove the list element at a specified index. The pop()
function returns the removed element. The following code example shows us how we can remove list elements by index with the pop()
function in Python.
list1 = [0, 1, 2, 3, 4]
removedElement = list1.pop(1)
print(list1)
print(removedElement)
Output:
[0, 2, 3, 4]
1
In the above code, we first initialize a list and then remove the element at index 1
of the list using the pop()
function.
Both del
keyword method and pop()
function perform the same task. The only difference is that the del
keyword deletes the element at the given index, but the pop()
function also returns the deleted element.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python