How to Remove Commas From String in Python
-
Remove Commas From String Using the
replace()
Method in Python -
Remove Commas From String Using the
re
Package in Python
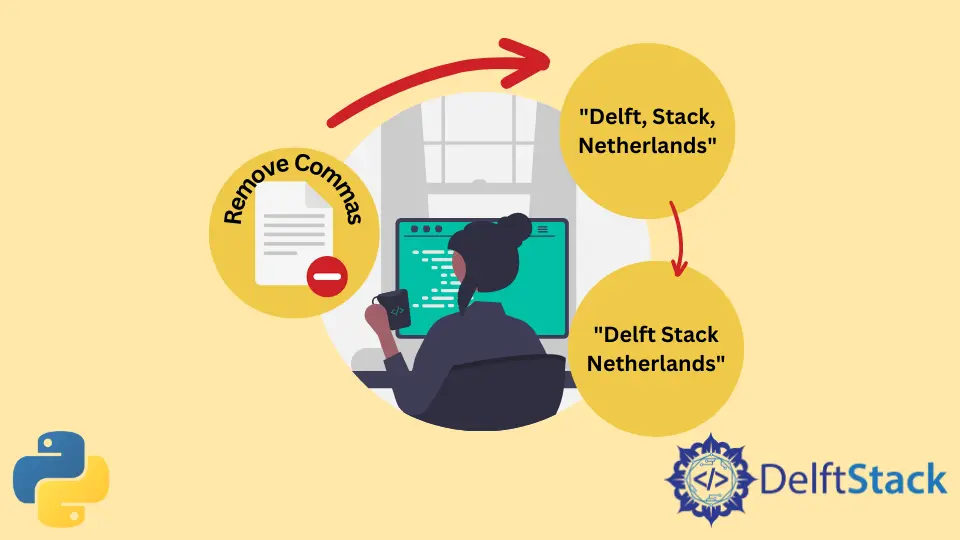
This tutorial explains how we can remove commas from a string using Python. To remove commas from a string in Python, we can use the replace()
method or the re
package.
We will use the string in the code snippet below to demonstrate how we can remove commas from a string in Python.
my_string = "Delft, Stack, Netherlands"
print(my_string)
Output:
Delft, Stack, Netherlands
Remove Commas From String Using the replace()
Method in Python
The replace()
method in Python str
class replaces a substring with the specified substring and returns the transformed string.
Syntax of replace()
Method:
str.replace(old, new, count)
Parameters
old |
substring, which is to be replaced in the string str |
new |
substring used to replace old substring in the string str |
count |
optional parameter that specifies how many times old is replaced by new . If count is not provided, the method will replace all old substrings with new substring. |
Return
String in which old
substring is replaced by new
substring.
Example: Remove Commas From String Using the str.replace()
Method
my_string = "Delft, Stack, Netherlands"
print("Original String is:")
print(my_string)
transformed_string = my_string.replace(",", "")
print("Transformed String is:")
print(transformed_string)
Output:
Original String is:
Delft, Stack, Netherlands
Transformed String is:
Delft Stack Netherlands
It replaces all the commas in the string my_string
with ""
. Hence, all the ,
in the string my_string
are removed.
If we only wish to remove the first ,
in the my_string
, we can do so by passing the count
parameter in the replace()
method.
my_string = "Delft, Stack, Netherlands"
print("Original String is:")
print(my_string)
transformed_string = my_string.replace(",", "", 1)
print("Transformed String is:")
print(transformed_string)
Output:
Original String is:
Delft, Stack, Netherlands
Transformed String is:
Delft Stack, Netherlands
As the value of count is set to 1 in the replace()
method, it only removes the first comma in the string my_string
.
Remove Commas From String Using the re
Package in Python
In the re
pacakge of Python, we have sub()
method, which can also be used to remove the commas from a string.
import re
my_string = "Delft, Stack, Netherlands"
print("Original String is:")
print(my_string)
transformed_string = re.sub(",", "", my_string)
print("Transformed String is:")
print(transformed_string)
Output:
Original String is:
Delft, Stack, Netherlands
Transformed String is:
Delft Stack Netherlands
It replaces all the ,
in the string my_string
with ""
and removes all the commas in the string my_string
.
The first argument to the re.sub()
method is substring to be replaced, the second argument is the substring to replace with, and the third argument is the string in which replacement is to be done.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn