How to Remove Certain Characters From String in Python
-
Remove Certain Characters From String in Python Using the
string.replace()
Method -
Remove Certain Characters From String in Python Using the
string.join()
Method -
Remove Certain Characters From String in Python Using the
re.sub()
Method
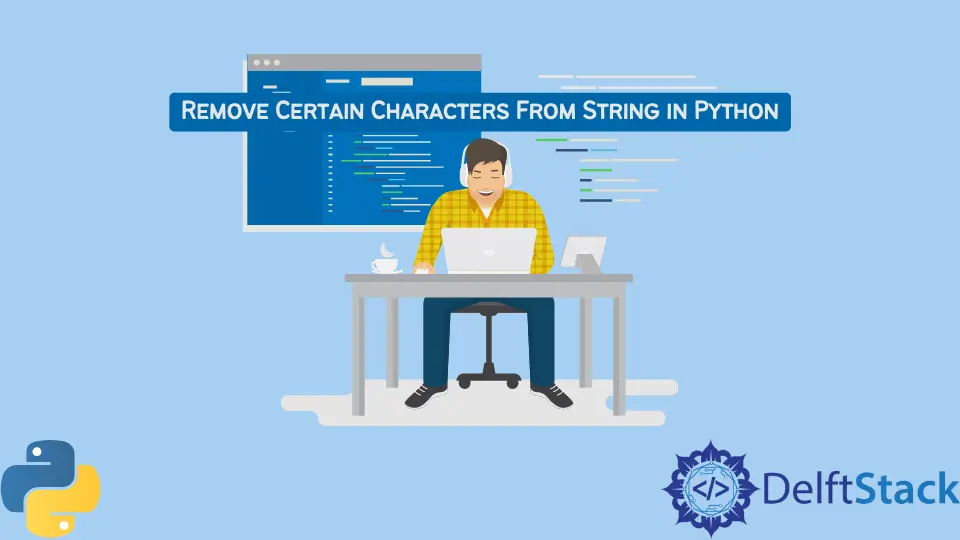
This tutorial will explain various methods to remove certain characters from a string in Python. In many cases, we need to remove punctuation marks or a certain special character from the text, like for data cleaning.
Remove Certain Characters From String in Python Using the string.replace()
Method
The string.replace()
method returns a new string after replacing the first string argument with the second string argument. To remove certain characters from the string using the string.replace()
method, we can use the for
loop to remove one character per iteration from a string.
As we want to remove the characters and not replace them, we will pass an empty string as the second argument. The below example code demonstrates how to remove characters from the string using the string.replace()
method.
string = "Hey! What's up?"
characters = "'!?"
for x in range(len(characters)):
string = string.replace(characters[x], "")
print(string)
Output:
Hey Whats up
Remove Certain Characters From String in Python Using the string.join()
Method
The string.join(iterable)
method joins each element of the iterable
object with the string
and returns a new string. To remove certain characters from the string using the string.join()
method, we will have to iterate through the whole string and drop the characters we need to remove from the string. The below example code demonstrates how we can do it in Python using string.join()
.
string = "Hey! What's up?"
characters = "'!?"
string = "".join(x for x in string if x not in characters)
print(string)
Output:
Hey Whats up
Remove Certain Characters From String in Python Using the re.sub()
Method
The re.sub(pattern, repl, string, count)
method of the re
module returns a new string after replacing the regular expression pattern
with the value of repl
in the original string. The count
means the number of times we want to replace the pattern
from the string.
As we need to remove but not replace any character, the repl
will be equal to an empty string. The below code example demonstrates how we can use the re.sub()
method to replace characters from the string in Python.
import re
string = "Hey! What's up?"
string = re.sub("\!|'|\?", "", string)
print(string)
Output:
Hey Whats up