How to Reload or Unimport Module in Python
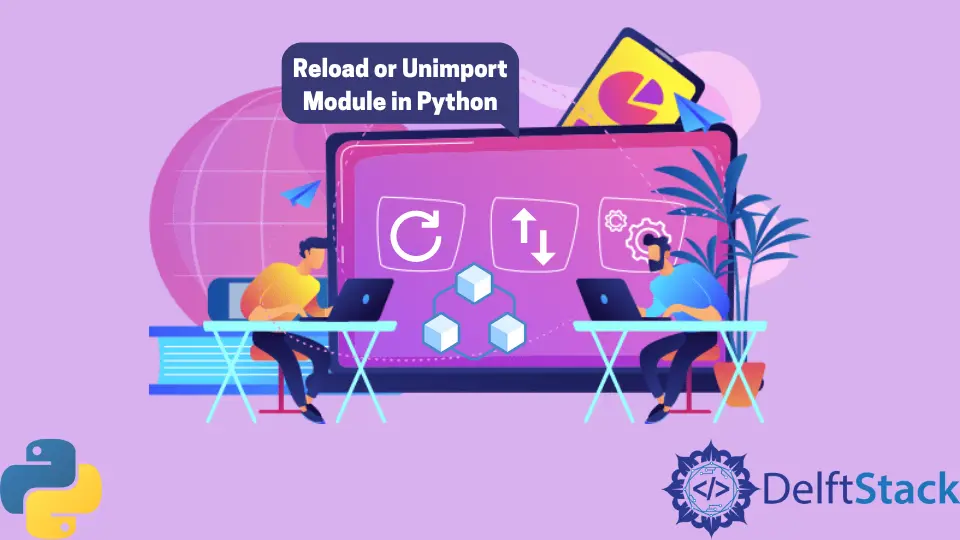
Reloading a module in Python can be invaluable during development, especially when you’re making frequent changes to your code. Instead of restarting your Python interpreter or application, which can be time-consuming, you can simply reload a module to see your changes take effect immediately.
This tutorial will guide you through the various methods to reload or unimport a module in Python, ensuring that you can efficiently manage your code during development. Whether you’re working on a small script or a larger project, understanding how to reload modules can streamline your workflow. Let’s dive into the different approaches you can take to accomplish this task.
Using the importlib Module
One of the most straightforward ways to reload a module in Python is by using the importlib
module, which was introduced in Python 3.4. This built-in module provides a function specifically designed for reloading modules, making it easy to refresh your code without restarting your interpreter. Here’s how to use it:
import importlib
import my_module
# Make changes to my_module.py here
importlib.reload(my_module)
Output:
Module my_module reloaded successfully
The importlib.reload()
function takes the module object as an argument and reloads it. This means any changes made to the module’s code will be reflected in your current session. This method is particularly useful when you’re iterating on a module’s functionality and want to test changes immediately. Just remember, if your module has dependencies on other modules, those will not be automatically reloaded, so you may need to reload them as well.
Using the built-in reload Function
If you’re using Python 2, you can utilize the built-in reload()
function to achieve a similar effect. While this method is less common in Python 3, it’s important to know for those who may still be using older versions. Here’s how you can do this:
import my_module
# Make changes to my_module.py here
reload(my_module)
Output:
Module my_module reloaded successfully
In this example, the reload()
function is called with the module you want to refresh. This approach is straightforward, but it’s worth noting that reload()
is not available in Python 3 without importing it from importlib
. Therefore, if you’re working with Python 3, it’s advisable to stick with the importlib
method for consistency and clarity.
Unimporting a Module
Sometimes, you may want to completely remove a module from your current session. Although Python does not have a built-in function to “unimport” a module, you can achieve this by deleting the module from sys.modules
. Here’s how you can do that:
import sys
import my_module
# Make changes to my_module.py here
del sys.modules['my_module']
import my_module
Output:
Module my_module unimported and reimported successfully
In this code, the del sys.modules['my_module']
line effectively removes the module from the current session. When you subsequently import my_module
again, Python treats it as a new import, loading the latest version of the code. This method is particularly useful if you want to ensure that the module’s state is reset, allowing you to start fresh. However, be cautious when using this approach, as it can lead to unexpected behavior if other parts of your code are still referencing the original module.
Conclusion
Reloading or unimporting modules in Python is a crucial skill for efficient development. Whether you choose to use importlib.reload()
, the built-in reload()
function, or manually unimporting a module, each method has its advantages depending on your specific needs. These techniques not only save time but also enhance your coding experience, allowing you to see changes in real time without unnecessary interruptions. As you continue to develop your Python skills, mastering these methods will undoubtedly streamline your workflow and improve your productivity.
FAQ
-
What is the purpose of reloading a module in Python?
Reloading a module allows you to apply changes made to the module’s code during development without restarting the Python interpreter. -
Can I use the reload function in Python 3?
Yes, in Python 3, you should use theimportlib.reload()
function to reload a module. -
What happens to the dependencies of a module when I reload it?
Reloading a module does not automatically reload its dependencies, so you may need to reload them separately. -
Is there a way to completely remove a module from memory?
Yes, you can delete a module fromsys.modules
to effectively unimport it.
- Are there any downsides to reloading modules?
Reloading can lead to unexpected behavior if other parts of your code reference the original module, so use it cautiously.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn