How to Implement Recursive Multiplication in Python
Fumbani Banda
Feb 02, 2024
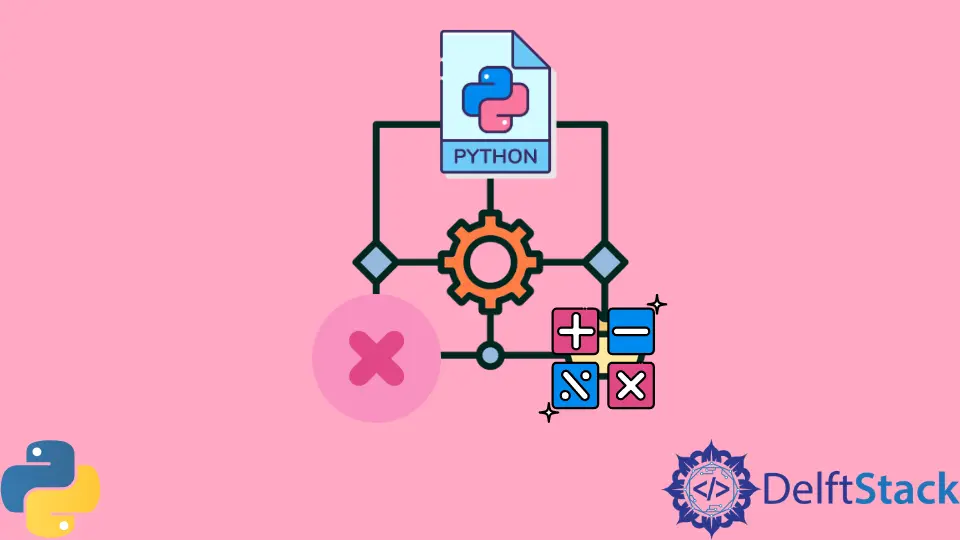
This tutorial will introduce integer multiplication with recursion in Python.
Recursion is a technique that uses a function that calls itself one or more times until a base condition is met, at which time the rest of the repetition is processed from the last one called to the first. Recursion is widely used in programming to solve complex problems where the solution depends on the solutions to smaller instances of the same problem.
Recursive Multiplication in Python
Multiplication of a number is repeated addition. Recursive multiplication would repeatedly add the larger number of the two numbers, (x,y)
to itself until we get the required product.
Assume that x >= y
. Then we can recursively add x
to itself y
times. In this case, you recursively add 3 to itself twice.
def multiply(x, y):
if y == 0:
return 0
elif y < 0:
return -(x - multiply(x, y + 1))
else:
return x + multiply(x, y - 1)
if __name__ == "__main__":
print("3 * 2 = ", multiply(3, 2))
print("3 * (-2) = ", multiply(3, -2))
print("(-3) * 2 = ", multiply(-3, 2))
print("(-3) * (-2)= ", multiply(-3, -2))
Output:
3 * 2 = 6
3 * (-2) = -6
(-3) * 2 = -6
(-3) * (-2)= 6
Author: Fumbani Banda