How to Open a Zip File Without Extracting It in Python
-
Use the
zipfile.ZipFile()
Function to Open a Zip File Without Temporarily Extracting It in Python -
Use the
ZipFile.open()
Function to Open a Zip File Without Temporarily Extracting It in Python
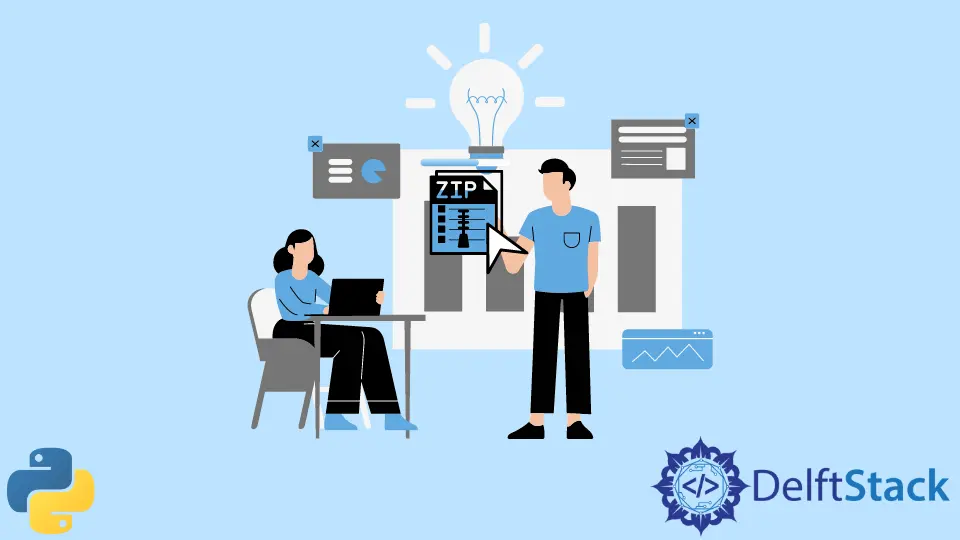
This article explains how to open a zip file without temporarily extracting it in the Python software. To open a zip file without temporarily extracting it in Python, use the zipfile
Python library.
For this, import the zipfile
standard library. Then, use either of the following functions.
- Use the
zipfile.ZipFile()
function in read-mode. - Use the
ZipFile.open()
function in read-mode.
Before we begin, please get the zip file ready. Follow the below steps.
-
Prepare a text file named
mail.txt
with some content inside it as:This is from mail.txt
-
Zip the
mail.txt
file. -
Name the zip file as
mail.zip
.
Use the zipfile.ZipFile()
Function to Open a Zip File Without Temporarily Extracting It in Python
Below is an example program that shows you how to open a zip file without temporarily extracting it in Python. Use the zipfile.ZipFile()
function in read-mode in the following way.
zipfile.ZipFile(file, mode="r")
Here, the file
is either:
- A path to a file (a string)
- A file-like object
- A path-like object
For example,
import zipfile
archive = zipfile.ZipFile("mail.zip", "r")
# Let us verify the operation..
txtdata = archive.read("mail.txt")
print(txtdata)
Output:
b'This is from mail.txt'
Use the ZipFile.open()
Function to Open a Zip File Without Temporarily Extracting It in Python
Here’s an example that demonstrates how to open a zip file without temporarily extracting it in Python.
Here, we use the open()
function in read-mode.
ZipFile.open(name, mode="r")
The member of the zip file is treated as a binary file-like object. The name
here can be either:
- The name of a file within the zip
- A
ZipInfo
object
Here’s an example.
import zipfile
with zipfile.ZipFile("mail.zip") as thezip:
with thezip.open("mail.txt", mode="r") as thefile:
# Let us verify the operation..
print(thefile.read())
Output:
b'This is from mail.txt'