How to Read Matlab mat Files in Python
-
Use the
scipy.io
Module to Read.mat
Files in Python -
Use the
NumPy
Module to Readmat
Files in Python -
Use the
mat4py
Module to Readmat
Files in Python -
Use the
matlab.engine
Module to Readmat
Files in Python
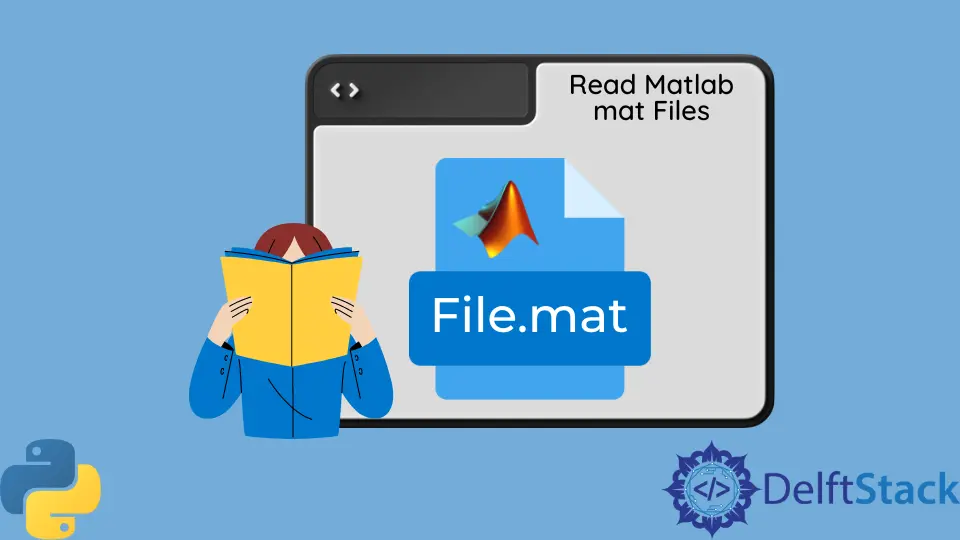
MATLAB is a programming platform that is widely used these days for numerical computation, statistical analysis, and generating algorithms. It is a very flexible language and allows us to integrate our work with different programming languages like Python.
The MATLAB workspace saves all its variables and contents in a mat
file. In this tutorial, we will learn how to open and read mat
files in Python.
Use the scipy.io
Module to Read .mat
Files in Python
The scipy.io
module has the loadmat()
function, which can open and read mat
files. The following code shows how to use this function.
import scipy.io
mat = scipy.io.loadmat("file.mat")
Note that this method does not work for the MATLAB version below 7.3. We can either save the mat
file in lower versions using the below command in MATLAB to avoid this.
save('test.mat', '-v7')
Use the NumPy
Module to Read mat
Files in Python
It is discussed earlier how we cannot open files in MATLAB 7.3 using the scipy.io
module in Python. It is worth noting that files in version 7.3 and above are hdf5 datasets, which means we can open them using the NumPy
library. For this method to work, the h5py
module needs to be installed, which requires HDF5 on your system.
The code below shows how to read mat
files using this method.
import numpy as np
import h5py
f = h5py.File("somefile.mat", "r")
data = f.get("data/variable1")
data = np.array(data) # For converting to a NumPy array
Use the mat4py
Module to Read mat
Files in Python
This module has functions that allow us to write and read data to and from MATLAB files.
The loadmat()
function reads MATLAB files and stores them in basic Python structures like a list or a dictionary and is similar to the loadmat()
from scipy.io
.
For example,
from mat4py import loadmat
data = loadmat("example.mat")
Use the matlab.engine
Module to Read mat
Files in Python
For users who already have MATLAB can use the matlab.engine
which is provided by MathWorks itself. It has a lot of functionality, which extends to more than just reading and writing “.mat” files.
The following code shows how to read MATLAB files using this method.
import matlab.engine
eng = matlab.engine.start_matlab()
content = eng.load("example.mat", nargout=1)
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn