How to Read File to a String in Python
-
Use the
read()
Method to Read a Text File to a String in Python -
Use the
pathlib.read_text()
Function to Read a Text File to a String in Python -
Use the
join()
Function to Read a Text File to a String in Python
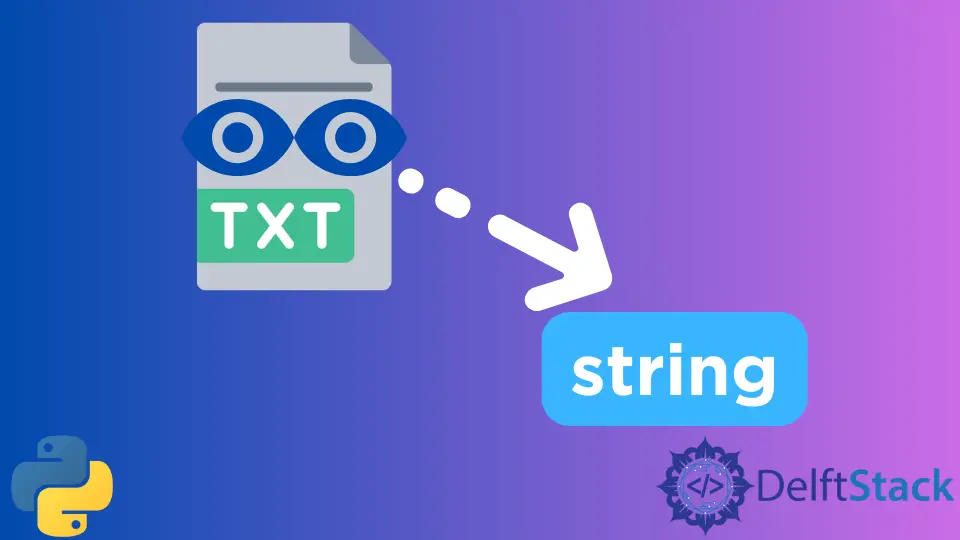
In Python, we have built-in functions that can handle different file operations on different file types. A text file contains a sequence of strings in which every line terminated using a newline character \n
.
In this tutorial, we will learn how to read a text file into a string in Python.
Use the read()
Method to Read a Text File to a String in Python
The read()
method of the file object allows us to read all the content from a text file all at once. First, we will create a file-object and open the required text file in reading mode using the open()
function. Then we will use the read()
function with this file-object to read all text into a string and print it as shown below.
with open("sample.txt") as f:
content = f.read()
print(content)
Output:
sample line 1\n sample line 2\n sample line 3\n
When we read a file, it reads the newline character \n
as well. We can remove this character using the replace()
function. This function will replace all newline characters from the string with a specified character in the function.
For example,
with open("sample.txt") as f:
content = f.read().replace("\n", " ")
print(content)
Output:
sample line 1 sample line 2 sample line 3
Use the pathlib.read_text()
Function to Read a Text File to a String in Python
The pathlib
module is added to Python 3.4 and has more efficient methods available for file-handling and system paths. The read_text()
function from this module can read a text file and close it in the same line. The following code shows this.
from pathlib import Path
content = Path("sample.txt").read_text().replace("\n", " ")
print(content)
Output:
sample line 1 sample line 2 sample line 3
Use the join()
Function to Read a Text File to a String in Python
The join()
method allows us to join different iterables in Python. We can read a text file into a string using this function also. For this, we will read everything using the file-object and then use the list comprehension method and combine them using the join()
function. The code below implements this.
with open("sample.txt") as f:
content = " ".join([l.rstrip() for l in f])
print(content)
Output:
sample line 1 sample line 2 sample line 3
The rstrip()
function here removes any trailing characters from the line.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python File
- How to Get All the Files of a Directory
- How to Append Text to a File in Python
- How to Check if a File Exists in Python
- How to Find Files With a Certain Extension Only in Python
- How to Read Specific Lines From a File in Python
- How to Check if File Is Empty in Python