How to Read Specific Column From .dat File in Python
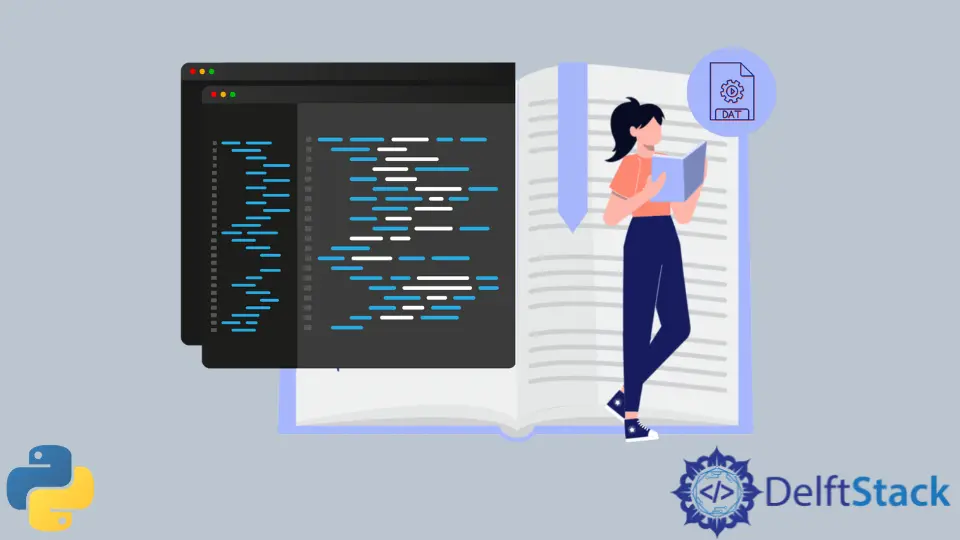
We have a huge data storage containing multiple data in a single file when working with big data. In many cases working with big data, we are not interested in all columns and rows in that file. We only need specific rows and columns from that file to continue the business requirements. So, in this article, we will learn about methods known as extracting specific columns or rows from the .dat file
. However, there are various ways to do this in Python, and we will see some of them with examples below.
As we already know that the .dat file consists of generic data about any domain. The data sets consist of any type of information with the same data type. These formats are best used to represent sets or sequences of records in which each record has an identical list of fields.
Read Specific Column From .dat
File in Python
Let’s assume we have a .dat
file having the records of the prices, and we want to extract that particular column and do some analysis on it. First, we have to import the .dat
file using the Python built-in file open function. Then using the split()
function, we will extract the required column by passing it within the split() function parameter. It will, by default, extract the whole column for us. Then we will display the entire column on the screen using the print()
function in Python.
with open(r"airline.dat") as datFile:
print([data.split()[0] for data in datFile])
Output:
Year
1997
1999
1998
1996
Abdul is a software engineer with an architect background and a passion for full-stack web development with eight years of professional experience in analysis, design, development, implementation, performance tuning, and implementation of business applications.
LinkedIn