raw_input in Python 3
- What is raw_input and its Replacement in Python 3?
- Capturing Different Types of User Input
- Using Exception Handling with User Input
- Enhancing User Interaction with Input Prompts
- Conclusion
- FAQ
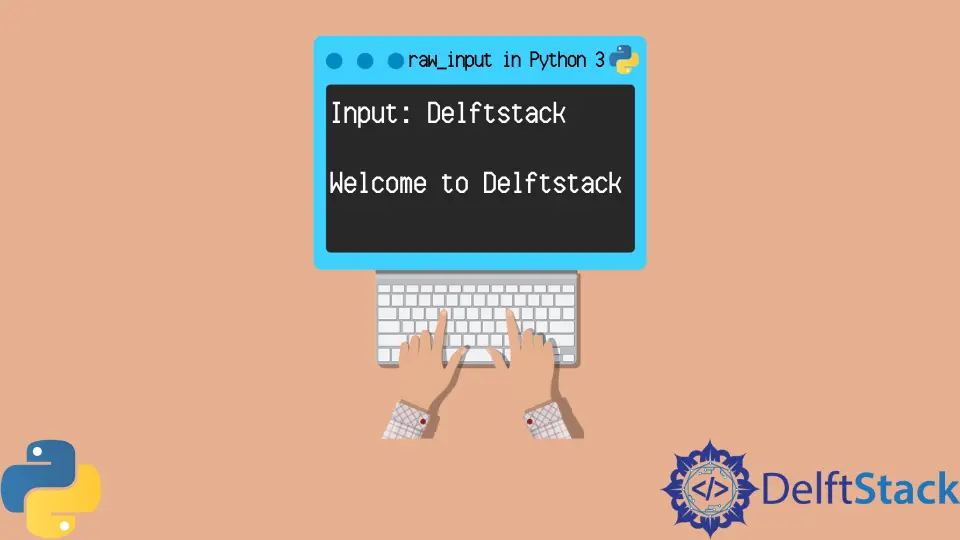
In the world of programming, user input is a fundamental aspect that can significantly enhance the interactivity of your applications. In Python 2, the raw_input()
function was the go-to method for capturing user input as a string. However, with the introduction of Python 3, this function was replaced by input()
.
This tutorial will explore how to effectively use input()
in Python 3, ensuring you can gather user data seamlessly. Whether you’re a beginner or someone looking to brush up on your skills, this guide will walk you through the essentials of user input in Python 3.0 and above, complete with practical examples and explanations.
What is raw_input and its Replacement in Python 3?
In Python 2, raw_input()
was used to read a line from input, returning it as a string. This was particularly useful for gathering user inputs in console applications. However, in Python 3, raw_input()
was removed and replaced with input()
, which behaves like raw_input()
from Python 2. This means that when you call input()
, it captures user input as a string, making it straightforward to interact with users.
Here’s a simple example to illustrate how input()
works in Python 3:
user_name = input("Enter your name: ")
print("Hello, " + user_name + "!")
When this code runs, it prompts the user to enter their name. Once they do, it greets them with a friendly message.
Output:
Enter your name: Alice
Hello, Alice!
In this snippet, the input()
function captures the user’s input and assigns it to the variable user_name
. The print()
function then uses that variable to display a personalized greeting. This straightforward interaction is the essence of using input()
in Python 3.
Capturing Different Types of User Input
While input()
captures everything as a string, you may often need to convert this input into other data types, such as integers or floats. Let’s look at how to do this effectively.
Here’s an example that captures a number from the user and performs a basic arithmetic operation:
number = input("Enter a number: ")
number = int(number)
result = number * 2
print("Double your number is: " + str(result))
In this example, the user is prompted to enter a number. The input is initially a string, so we convert it to an integer using int()
. We then multiply the number by two and print the result.
Output:
Enter a number: 5
Double your number is: 10
This method showcases how to handle various data types effectively. By converting the input, you can perform arithmetic operations and manipulate the data as needed. It’s essential to ensure that the user inputs a valid number; otherwise, you may encounter a ValueError
. To enhance user experience, consider implementing error handling.
Using Exception Handling with User Input
User input can be unpredictable, and it’s crucial to handle potential errors gracefully. By using exception handling, you can ensure that your program doesn’t crash when a user provides invalid input. Here’s how you can implement it:
while True:
try:
number = input("Enter a number: ")
number = int(number)
break
except ValueError:
print("That's not a valid number. Please try again.")
result = number * 2
print("Double your number is: " + str(result))
In this code, we use a while
loop to continuously prompt the user until they provide a valid integer. The try
block attempts to convert the input into an integer, while the except
block catches any ValueError
that arises from invalid input. This way, the user receives feedback and can correct their input without crashing the program.
Output:
Enter a number: abc
That's not a valid number. Please try again.
Enter a number: 4
Double your number is: 8
This method not only improves user experience but also ensures that your program remains robust and user-friendly. By incorporating exception handling, you can manage errors effectively and keep your application running smoothly.
Enhancing User Interaction with Input Prompts
The way you prompt users for input can significantly affect their experience. A well-structured prompt can guide users on what kind of input is expected. Here’s an example that demonstrates how to provide clear instructions:
age = input("Please enter your age (in years): ")
age = int(age)
if age < 18:
print("You are a minor.")
else:
print("You are an adult.")
In this example, the prompt clearly states what the user should enter. After capturing the input, the program checks whether the user is a minor or an adult based on their age.
Output:
Please enter your age (in years): 21
You are an adult.
Providing clear and concise prompts can significantly enhance user interaction. It reduces confusion and helps users understand exactly what is expected, leading to a smoother experience.
Conclusion
Understanding how to use input()
in Python 3 is essential for creating interactive applications. By capturing user input effectively, converting data types, handling exceptions, and crafting clear prompts, you can significantly enhance the usability of your programs. Whether you’re building a simple console application or a more complex system, mastering user input will empower you to create engaging and user-friendly experiences. Embrace these techniques, and watch your Python projects come to life!
FAQ
-
What is the difference between raw_input and input in Python?
raw_input was used in Python 2 to capture user input as a string, while input in Python 3 serves the same purpose but is the only function available for this task. -
Can I use input to capture numbers in Python 3?
Yes, input captures everything as a string, so you need to convert it to an integer or float using int() or float() for numerical operations. -
How do I handle invalid input in Python?
You can use exception handling (try-except blocks) to catch errors like ValueError when users enter invalid data. -
Is it necessary to convert input data types?
It’s necessary if you need to perform operations that require specific data types, like arithmetic operations on numbers.
- How can I improve user prompts in my Python programs?
Providing clear and concise prompts that specify the expected input format can significantly enhance user experience.