How to Solve Quadratic Equations in Python
-
Import the
math
Library in Python - Calculate the Discriminant Value to Solve Quadratic Equation in Python
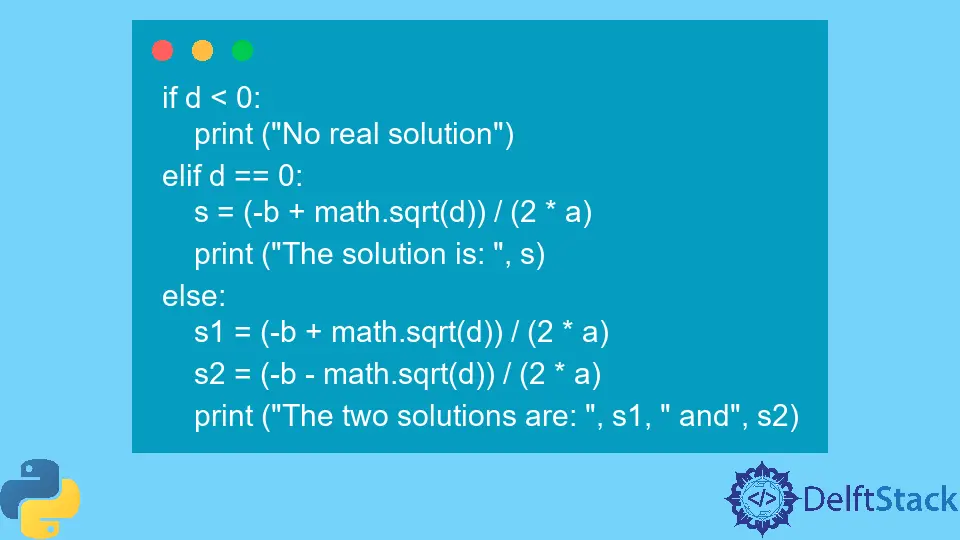
This tutorial demonstrates how to solve quadratic equations in Python.
Import the math
Library in Python
We must import the math
library to get started.
import math
We will take the three coefficients of a quadratic equation to solve the equation.
a = 1
b = 5
c = 6
Calculate the Discriminant Value to Solve Quadratic Equation in Python
We will now use the above three coefficient values to calculate the value of our discriminant. The formula to calculate the discriminant value is shown in the code below.
d = b ** 2 - 4 * a * c
We now have the value of our discriminant to solve the equation. Based on the value of the discriminant, we can divide our problem into three cases.
If the value of d
is less than zero
, we do not have a real solution if the value is exactly equal to zero
, we have only one solution, and if the value is greater than zero
, we will have 2 solutions for our equation. We code this as below.
if d < 0:
print("No real solution")
elif d == 0:
s = (-b + math.sqrt(d)) / (2 * a)
print("The solution is: ", s)
else:
s1 = (-b + math.sqrt(d)) / (2 * a)
s2 = (-b - math.sqrt(d)) / (2 * a)
print("The two solutions are: ", s1, " and", s2)
As seen above, we use if-else
to decide our solution based on the value of d
. We use math.sqrt()
function to calculate the square root of d
.
We get the below output when we run the above code with the sample coefficient values.
The two solutions are: -2.0 and -3.0
Since the value of d
in the sample case was 1. As seen above, we have two solutions: -2
and -3
.
Thus we have successfully learned how to solve a quadratic equation in Python.