How to Create a Zip Archive of a Directory Using Python
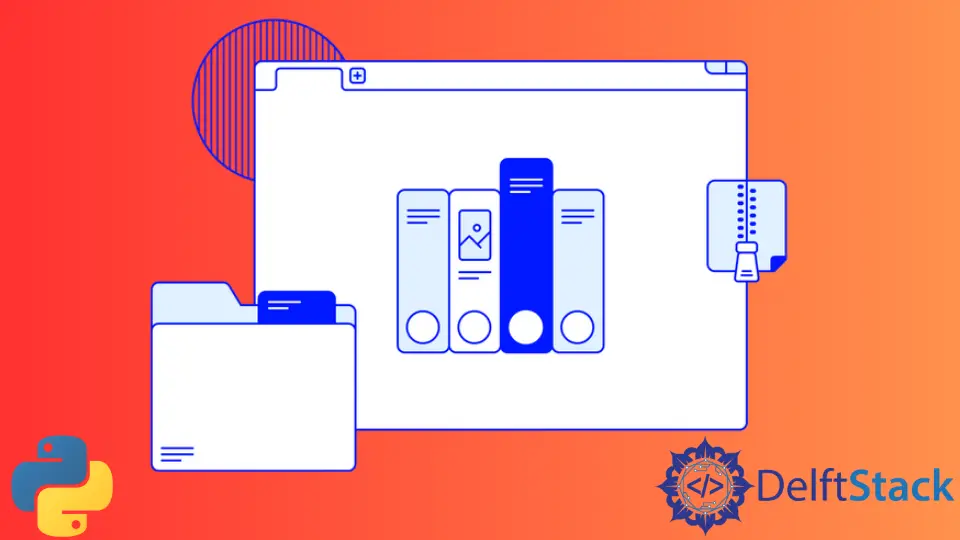
The zip file is an archive file format containing one or more compressed files. It supports lossless data compression and is the most commonly used archive format worldwide. This article will help us learn the ways to convert a directory to a zip file using the Python programming language.
Using the shutil
Library to Create a Zip Archive of a Directory
The shutil
library is a part of the standard Python. It offers several high-level functions to perform over files or groups of files, such as copying, compression, decompression, and removal. It has a function or method by the name of make_archive()
that can convert any directory or folder into a zip archive file. The following are the most used arguments of this function.
base_name
: Name of the file to be created along with the path and without any format extension.format
: It is the name of the format of the archive. The available options arezip
(requirezlib
module),tar
,gztar
(requirezlib
module),bztar
(requirebz2
module), andxztar
(requirelzma
module).root_dir
: It is the directory that will be the archive’s root directory, which means all the paths in the archive will be relative to it. The default value is the current directory.base_dir
: It is the directory from where archiving starts. Its value should be relative to theroot_dir
. The default value is the current directory.
Now that we are done with some brief theory about the package and the required function, let us learn how we can use this function to create a zip archive from a directory.
import os
import shutil
filename = "compressed"
format = "zip"
directory = os.getcwd()
shutil.make_archive(filename, format, directory)
The above code will compress the current working directory into a zip file and name the file to compressed.zip
. Note that we did not mention the extension (.zip
) in the base_name
.