How to Convert XML to JSON in Python
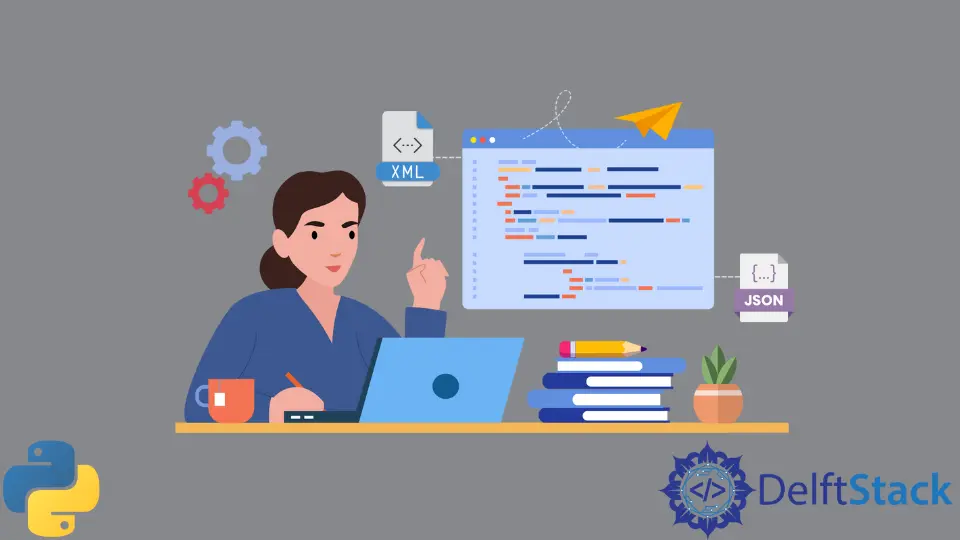
In this tutorial, we will look into the method to convert XML to JSON in Python. The JSON format is simpler to read and write than XML format; its compact style and lightweight also improve system performance, especially in RESTful APIs. As JSON is better than XML in many aspects, it is used as a replacement to XML in many fields.
Now, suppose we have data saved in XML format, and we want to convert it to JSON format; we can convert the XML data to JSON in Python using the following method.
Convert XML to JSON in Python Using the xmltodict
Module
In Python, we have the json.dumps(obj)
method that takes data as obj
argument and serializes it as the JSON formatted stream and returns the formatted data as output.
The obj
argument can be a dictionary, string, list, or tuple, etc, the json.dumps()
method converts the obj
according to its datatype. Like if we need a JSON object, we will have to provide the input as a dictionary, as the json.dumps()
method converts the dictionary to the JSON object. The list or tuple type is converted to JSON array and string type to JSON string.
We can use the xmltodict.parse()
method to convert the XML data to the Python dictionary data type. The xmltodict.parse()
method takes XML format data as a string, parses the data, and returns the output as a dictionary.
After converting the data to a dictionary using the xmltodict.parse()
method, we can use the json.dumps()
method to convert the data to the JSON object.
The below example code demonstrates how to convert the XML data to JSON using the json.dumps()
and xmltodict.parse()
method in Python.
import xmltodict
import json
dictionary = xmltodict.parse(
"""<note>
<date>2021-03-07</date>
<time>15:23</time>
<to>Sara</to>
<from>Khan</from>
<msg>Let's meet this weekend!</msg>
</note>"""
)
json_object = json.dumps(dictionary)
print(json_object)
Output:
{"note": {"date": "2021-03-07", "time": "15:23", "to": "Sara", "from": "Khan", "msg": "Let's meet this weekend!"}}
Related Article - Python XML
- How to Convert XML to CSV Using Python
- How to Pretty Print XML Output Pretty in Python
- How to Create an XML Parser in Python
- How to Convert XML to Dictionary in Python