How to Write to CSV Line by Line in Python
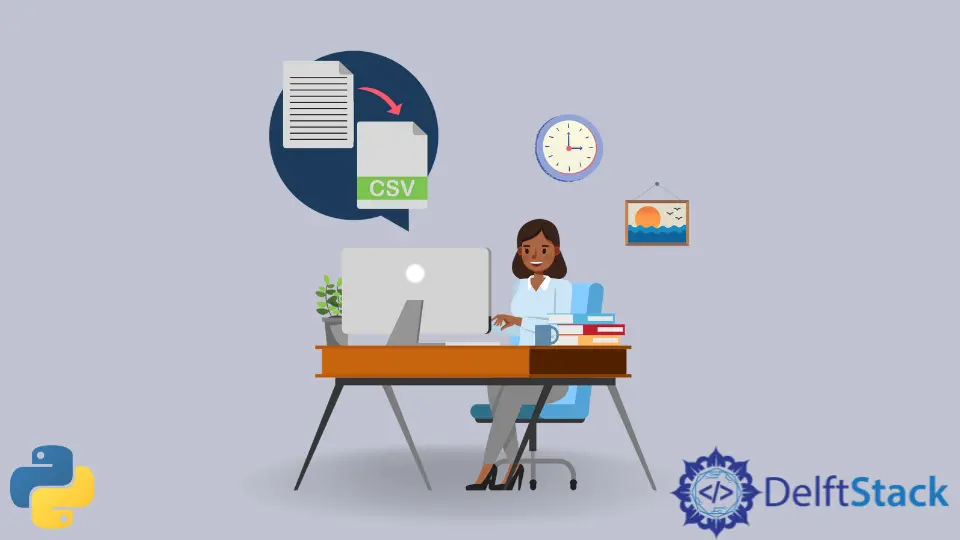
Writing data to CSV files is a common task in data handling and manipulation. Whether you’re exporting data from a database, logging information, or simply saving your work, knowing how to write to a CSV file in Python can be invaluable. There are two main methods to accomplish this: the traditional file handling method and the CSV writer method. Both approaches have their advantages, and understanding how to implement them can enhance your data processing skills.
In this article, we’ll explore both methods in detail, providing clear examples and explanations to help you master writing to CSV files line by line in Python.
Method 1: Traditional File Handling
The traditional file handling method involves using Python’s built-in file operations to write data to a CSV file. This method gives you a lot of control over how data is written, allowing you to customize the output format as needed. Here’s how you can do it:
data = [
['Name', 'Age', 'City'],
['Alice', 30, 'New York'],
['Bob', 25, 'Los Angeles'],
['Charlie', 35, 'Chicago']
]
with open('data.csv', 'w') as file:
for line in data:
file.write(','.join(map(str, line)) + '\n')
Output:
Name,Age,City
Alice,30,New York
Bob,25,Los Angeles
Charlie,35,Chicago
In this method, we first define our data as a list of lists, where each sublist represents a row in the CSV file. We open a file in write mode ('w'
) using the with
statement, which ensures that the file is properly closed after writing. Inside the loop, we convert each item in the sublist to a string (if necessary) and join them with commas. Finally, we append a newline character ('\n'
) to separate each row. This approach is straightforward and gives you the flexibility to format the output as you wish.
Method 2: Using the CSV Writer Module
The CSV module in Python provides a more structured way to handle CSV files. It automatically takes care of many of the intricacies involved in writing to CSV files, such as handling special characters and quoting. Here’s how to use the CSV writer:
import csv
data = [
['Name', 'Age', 'City'],
['Alice', 30, 'New York'],
['Bob', 25, 'Los Angeles'],
['Charlie', 35, 'Chicago']
]
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
Output:
Name,Age,City
Alice,30,New York
Bob,25,Los Angeles
Charlie,35,Chicago
In this method, we import the csv
module and use the csv.writer
function to create a writer object. We open the file in write mode ('w'
) and specify newline=''
to prevent extra blank lines on Windows. The writerows
method allows us to write multiple rows at once, which can be more efficient than writing them one by one. This approach is often preferred for its simplicity and reliability, especially when dealing with larger datasets or complex data structures.
Conclusion
Writing to CSV files in Python can be done effectively using either traditional file handling or the CSV writer module. Each method has its strengths, and the choice between them often depends on the specific requirements of your project. The traditional method offers more control over formatting, while the CSV module simplifies the process and reduces the chances of errors. By mastering both techniques, you can enhance your data manipulation capabilities and streamline your workflows.
FAQ
-
What is a CSV file?
A CSV file is a plain text file that contains data formatted as a table, with each line representing a row and each value separated by a comma. -
Why should I use the CSV module in Python?
The CSV module handles special characters and quoting automatically, reducing the risk of errors and making your code cleaner and more maintainable. -
Can I write to an existing CSV file without overwriting it?
Yes, you can open the file in append mode ('a'
) instead of write mode ('w'
) to add new data without deleting existing content. -
How can I read a CSV file in Python?
You can use the CSV module’scsv.reader
function to read a CSV file, which allows you to iterate over the rows easily. -
Are there any performance differences between the two methods?
For small datasets, the performance difference is negligible. However, for larger datasets, using the CSV module is generally more efficient and less error-prone.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn