How to Write List to CSV Columns in Python
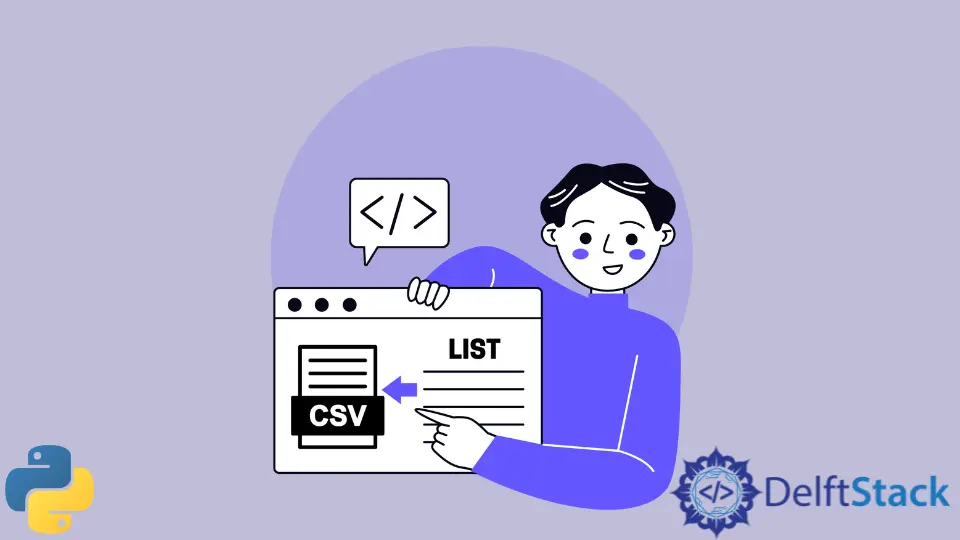
This tutorial demonstrates how to write a list to a CSV column in Python.
We will first create a sample CSV file in which we will add our list by the name Sample.csv
in a folder. In our case, we create the CSV file in the same location as our Python file.
Import the csv
Library in Python
We import the csv
library to work on the CSV file.
import csv
We will now create 5 sample lists to be added to the CSV file. We create them in the following way.
l1 = ["a", "b", "c", "d", "e"]
l2 = ["f", "g", "i", "j", "k"]
l3 = ["l", "m", "n", "o", "p"]
l4 = ["q", "r", "s", "t", "u"]
l5 = ["v", "w", "x", "y", "z"]
Zip All Lists in Python
We will now zip our 5 lists using the zip()
function and change them to rows.
r = zip(l1, l2, l3, l4, l5)
The above code will zip our 5 lists.
Add Elements to Columns in Python
We will now open our CSV using the open()
function and make our CSV file write-ready by using csv.writer()
function.
We write our list elements to our CSV file by taking individual elements and adding them into a column using the writerow()
function. We run the below code to add list elements into the columns.
with open("Sample.csv", "w") as s:
w = csv.writer(s)
for row in r:
w.writerow(row)
The above will result in the following output.
We can see that the list elements have been successfully added to the columns in our CSV file.
Thus using the above method, we can successfully write lists to a CSV column in Python.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python