How to Write Line by Line to a File Using Python
Vaibhav Vaibhav
Feb 02, 2024
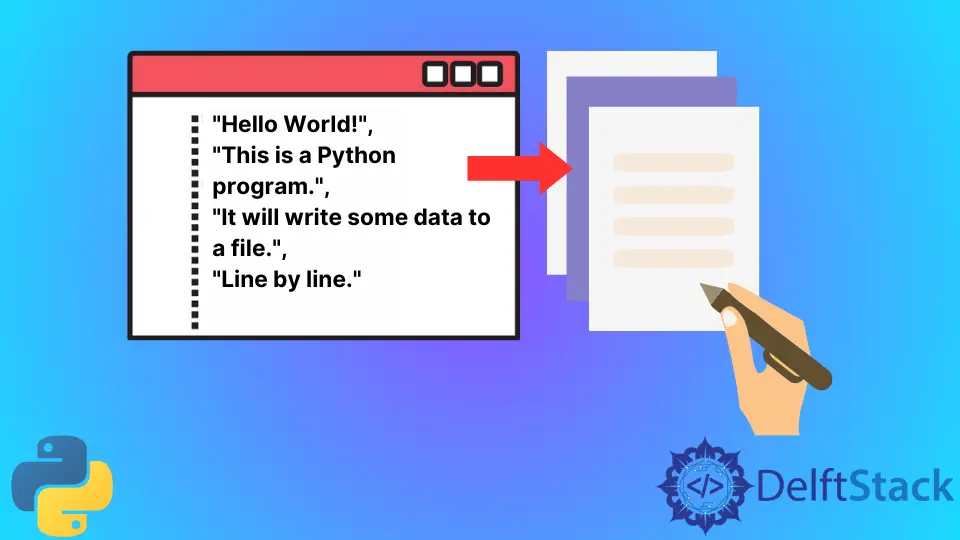
When learning to program, we must know how to work with files. We should know how to read data from a file, how to write data to a file, how to append data to a file, etc. This article will not focus on all the operations we can perform over files but learn how to write to a file line by line using Python.
Write to a File Line by Line Using Python
Suppose we have a bunch of strings that we have to write to a file. To write them line by line, we have to append an end-line character or \n
at the end of each line so that the strings appear individually. Refer to the following code for the same.
data = [
"Hello World!",
"This is a Python program.",
"It will write some data to a file.",
"Line by line.",
]
file = open("file.txt", "w")
for line in data:
file.write(line + "\n")
file.close()
Author: Vaibhav Vaibhav