How to Write Float Values to a File in Python
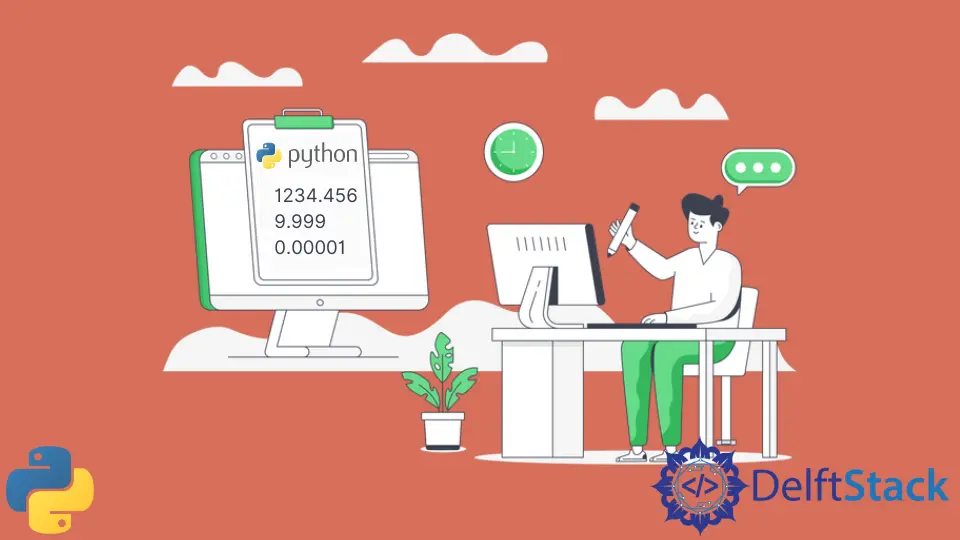
Python makes writing data to a file a seamless task. Data is written to a file in the form of strings. This article will learn how to float values to a file in Python.
Write Float Values to a File in Python
To write float values to a file in Python, we can use formatted strings. Formatted strings are special strings in Python that allow us to insert string representations of objects inside strings. Unlike regular strings, the formatted strings are prefixed with an f
. The following Python code depicts how to use the discussed approach.
data = [1234.342, 55.44257, 777.5733463467, 9.9999, 98765.98765]
f = open("output.txt", "w")
for d in data:
f.write(f"{d}\n")
f.close()
Output:
1234.342
55.44257
777.5733463467
9.9999
98765.98765
Apart from formatted strings, we can also use the in-built str()
method that returns string representations of objects. We can use this method to convert float values to their string equivalents and write them to a file. Refer to the following Python code for the same.
data = [1234.342, 55.44257, 777.5733463467, 9.9999, 98765.98765]
f = open("output.txt", "w")
for d in data:
f.write(str(d))
f.write("\n")
f.close()
Output:
1234.342
55.44257
777.5733463467
9.9999
98765.98765