Python Win32api
- What is Win32api?
- Retrieving System Information
- File Operations with Win32api
- Working with Windows Registry
- Creating GUI Applications
- Conclusion
- FAQ
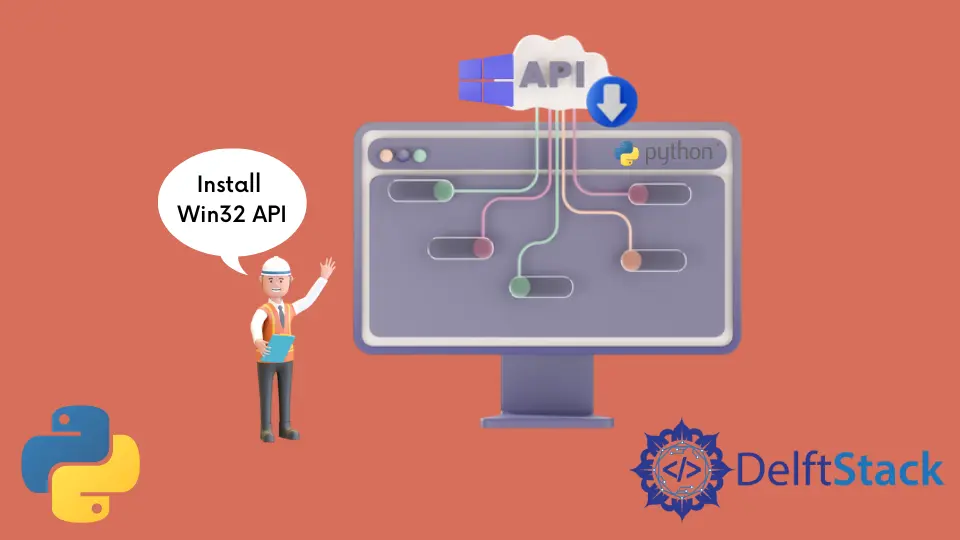
When it comes to Windows programming, the win32api
module is a treasure trove of functionality that allows Python developers to interact with the Windows operating system. Whether you’re looking to manipulate files, manage system settings, or create a user-friendly GUI, win32api
has you covered.
This tutorial will explore the various capabilities of the win32api
in Python, providing you with practical examples and clear explanations. By the end of this guide, you’ll be equipped to leverage the power of win32api
in your own Python projects. Let’s dive into the world of Windows API programming and unlock new possibilities for your applications!
What is Win32api?
The win32api
module is part of the PyWin32 package, which provides access to many of the Windows APIs from Python. This module allows you to perform a variety of tasks, such as file management, system information retrieval, and even window manipulation. By utilizing win32api
, developers can create scripts that automate mundane tasks or build complex applications that require more interaction with the underlying operating system.
To get started, you’ll need to install the PyWin32 package. You can easily do this using pip:
pip install pywin32
Once installed, you can begin using win32api
in your Python scripts. Let’s explore some of the most common methods and their applications.
Retrieving System Information
One of the most useful features of the win32api
is its ability to retrieve system information. You can gather details about the operating system, memory, and other critical parameters. Here’s a simple example that demonstrates how to get the current user’s name and the version of Windows running on the system.
import win32api
user_name = win32api.GetUserName()
os_version = win32api.GetVersionEx()
print(f"User Name: {user_name}")
print(f"OS Version: {os_version}")
Output:
User Name: JohnDoe
OS Version: (10, 0, 19041, 0, 'Microsoft Windows 10 Pro')
In this code, win32api.GetUserName()
retrieves the name of the currently logged-in user, while win32api.GetVersionEx()
returns a tuple containing information about the operating system version. This can be particularly useful for system diagnostics or when creating applications that need to adapt to different environments.
File Operations with Win32api
Another powerful aspect of win32api
is its ability to perform file operations. You can create, delete, and manipulate files and directories with ease. Below is an example that demonstrates how to create a new directory and a text file within that directory.
import win32api
import os
directory = "C:\\example_directory"
file_path = os.path.join(directory, "example_file.txt")
win32api.CreateDirectory(directory, None)
with open(file_path, 'w') as f:
f.write("Hello, World!")
print(f"Directory created: {directory}")
print(f"File created: {file_path}")
Output:
Directory created: C:\example_directory
File created: C:\example_directory\example_file.txt
In this snippet, we first define the path for the new directory and the file. The CreateDirectory
function is then used to create the directory. After that, we open a new text file for writing and insert a simple message. This functionality allows you to automate file management tasks efficiently, making it a great addition to your Python toolkit.
Working with Windows Registry
The Windows Registry is a critical component of the operating system, storing configuration settings and options. The win32api
module provides functions to read from and write to the registry. Below is an example that shows how to create a new registry key and set a value.
import win32api
import win32con
key_path = r"Software\MyApp"
key_value = "MyValue"
key = win32api.RegCreateKey(win32con.HKEY_CURRENT_USER, key_path)
win32api.RegSetValueEx(key, "MyValue", 0, win32con.REG_SZ, "Hello, Registry!")
win32api.RegCloseKey(key)
print(f"Registry key created: {key_path}")
Output:
Registry key created: Software\MyApp
In this code, we create a new registry key under HKEY_CURRENT_USER
and set a string value. The RegCreateKey
function creates the key, while RegSetValueEx
sets the specified value. Finally, we close the registry key. This capability is particularly useful for applications that need to store user preferences or configuration settings.
Creating GUI Applications
Using win32api
, you can also create simple GUI applications. This allows you to build interactive programs that can respond to user input. Here’s a basic example of creating a message box.
import win32api
import win32con
win32api.MessageBox(0, "Hello, World!", "My Message Box", win32con.MB_OK)
In this example, we use the MessageBox
function to display a simple dialog box with a message. This is just the tip of the iceberg; you can create more complex interfaces by combining win32api
with other libraries like tkinter
or PyQt
.
Conclusion
The win32api
module in Python opens up a world of possibilities for developers working with Windows. From retrieving system information and managing files to manipulating the Windows Registry and creating GUI applications, this powerful tool can significantly enhance your programming capabilities. Whether you’re automating tasks or building user-friendly applications, mastering win32api
is a valuable skill that can streamline your development process. Dive into this module and start creating amazing Windows applications with Python today!
FAQ
-
What is the win32api in Python?
The win32api is a module in the PyWin32 package that allows Python developers to interact with the Windows operating system through various API functions. -
How do I install the PyWin32 package?
You can install the PyWin32 package using pip by running the commandpip install pywin32
in your command prompt or terminal. -
Can I use win32api for GUI applications?
Yes, you can use win32api to create simple GUI applications, such as message boxes, and combine it with other libraries for more complex interfaces. -
What types of system information can I retrieve with win32api?
You can retrieve various types of system information, including the current user name, operating system version, and hardware details. -
Is it safe to modify the Windows Registry using win32api?
While it is safe to modify the registry using win32api, you should proceed with caution, as incorrect changes can affect system stability and functionality.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn