What Is the Python Wildcard
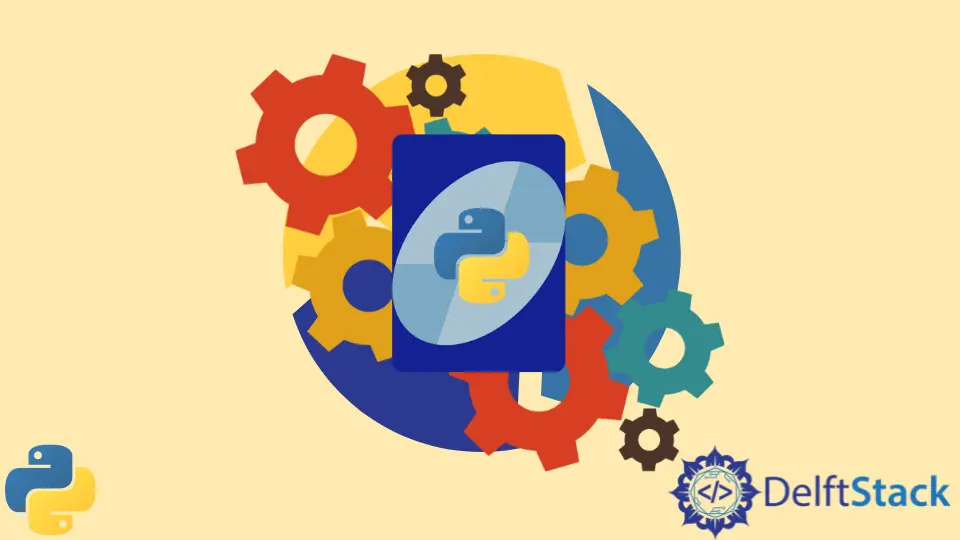
This tutorial will discuss what a wildcard is and how to do a wildcard search in Python.
Wildcard in Python
A wildcard can be described as a symbol utilized to act as an alias or replace one or more characters. The main cause of utilizing wildcards is to simplify searching criteria.
Most of its usage is predominantly in search engines, languages, operating systems, and computer programs. The asterisk *
and the question mark ?
are popular wildcards.
Types of Wildcards in Python
the Asterisk *
Wildcard in Python
The *
character or the asterisk can specify any number of characters. The asterisk *
is mostly utilized at the end of the given root word and when there is a need to search for endings with several possibilities for the given root word.
For example, if we take the word game*
, the search results for all possible outcomes would include both the words gamer
and games
. There would be other words along with these two, depending on the searching criteria and other words.
the Question Mark ?
Wildcard in Python
The question mark or the ?
character represents just one. It is utilized anywhere among the characters of the given root word. When a single word might contain several different spellings, the question mark operator makes things a bit easy.
The dot or the .
character is used for the single character representation instead of the question mark wildcard.
For example, if we take the word hon?r
, it would give out the result as honor
while ignoring honour
in this regard.
Wildcard Search in Python
The re
library needs to be imported to the Python code to implement wildcard search in Python. The re
library, which is an abbreviation of the term Regular Expression
, is a library that is utilized to deal with Regular Expressions
in Python.
We will create a list of words to perform the search operation and then use the re
library functions. We will find a match with a proper word with the help of wildcards.
The following code performs a wildcard search in Python.
import re
see = re.compile("hel.o")
x = ["hello", "welcome", "to", "delft", "stack"]
matches = [string for string in x if re.match(see, string)]
print(matches)
Output:
['hello']
Here, we took the dot (.)
wildcard to represent a single character wildcard throughout the search for finding the match of the given root word.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn