How to Get User Input in Python While Loop
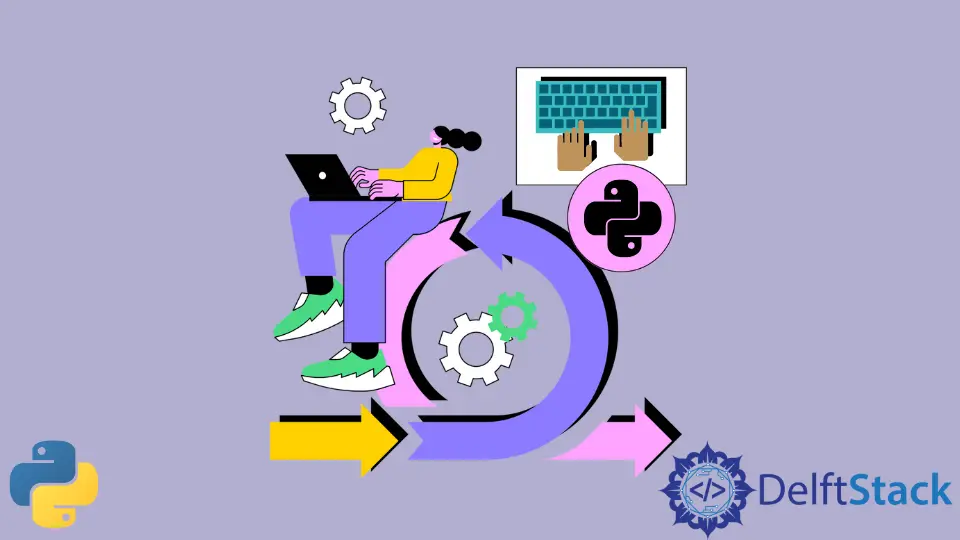
This tutorial will discuss the methods to get inputs from user multiple until a certain condition becomes true in Python.
User Input Inside a while
Loop in Python3
If we want to keep asking for input from the user until they input the required value, we can use the input()
function inside a while
loop.
In programming, there are two types of loops, counter-controlled and sentinel-controlled. In counter-controlled loops, we specify the number of times we want to execute the loop, while in sentinel-controlled loops, we specify a condition that needs to hold "true"
for the loop to execute.
The for
loop is a counter-controlled loop, meaning we have to specify how many times the loop will run before its execution.
The while
loop is a sentinel-controlled loop which means it will keep executing until a certain condition is satisfied.
To do this, we have to initialize our variable outside the loop. The following code snippet demonstrates how we can use an input()
function inside a while
loop.
Example Code:
name = "not maisam"
while name != "maisam":
name = input("please enter your name: ")
print("you guessed it right")
Output:
please enter your name: 123
please enter your name: abc
please enter your name: maisam
you guessed it right
The code in the above section will keep asking the user to input data until the user inputs maisam
.
User Input Inside a while
Loop in Python2
Unfortunately, the solution mentioned above fails in python2.
For this, we have to replace our input()
function with the raw_input()
function. It takes the user input and returns the result by removing the last \n
from the input.
This [raw_input()
function](raw_input — Python Reference (The Right Way) 0.1 documentation) in python2 is equivalent to the input()
function in python3. The following code example shows how we can use a raw_input()
function inside a while
loop.
Example Code:
name = "not maisam"
while name != "maisam":
name = raw_input("please enter your name: ")
print "you guessed it right"
Output:
please enter your name: 123
please enter your name: abc
please enter your name: maisam
you guessed it right
The code in the above section works the same way as the previous example and will keep asking the user to input data until the user inputs maisam
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn