How to Wait for Input in Python
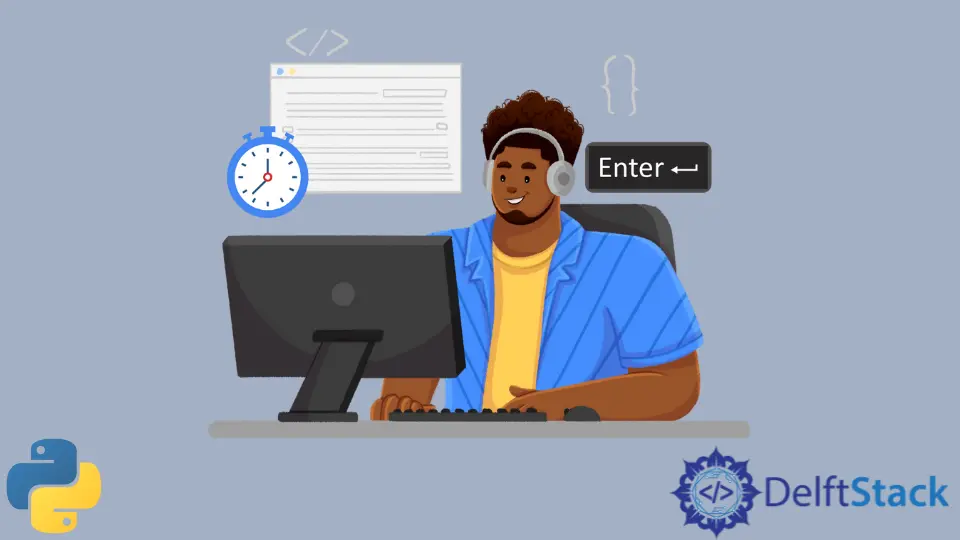
This tutorial shows you how to wait for key press in Python before proceeding with other operations.
Use input()
to Wait for Input in Python
input()
is a Python function that allows user input to be processed within the code. It temporarily halts all processes within the code therefore acts as a stopper to operations that are done.
In our case, we can use input()
as a key-listener to stop processes until the user presses a certain key. In the case of using input()
, user needs to press enter or return key.
Below is the example code.
def operation1(param):
# insert code here
pass
def operation2(param):
# insert code here
pass
def operation3(param):
# insert code here
pass
input("Press enter to start operations...")
ret = operation1("Sample Param")
print("\nOperation 1 has been executed successfully.")
input("\n Press enter to start operation 2...")
ret = operation2(ret)
print("Operation 2 has been executed successfully.")
input("\n Press enter to start final operation")
ret = operation3(ret)
print("All operations executed successfully. Returned a value of ", ret)
This way, before operations start, user needs to press Enter. Also, each subsequent operation needs user to press Enter as well, essentially adding a breather between the 3 operations.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn