How to Fix TypeError: Can Only Concatenate Tuple (Not Int) to Tuple
- Create a Tuple With a Single Object
-
Fix the
TypeError: Can Only Concatenate Tuple (Not "Int") To Tuple
in Python
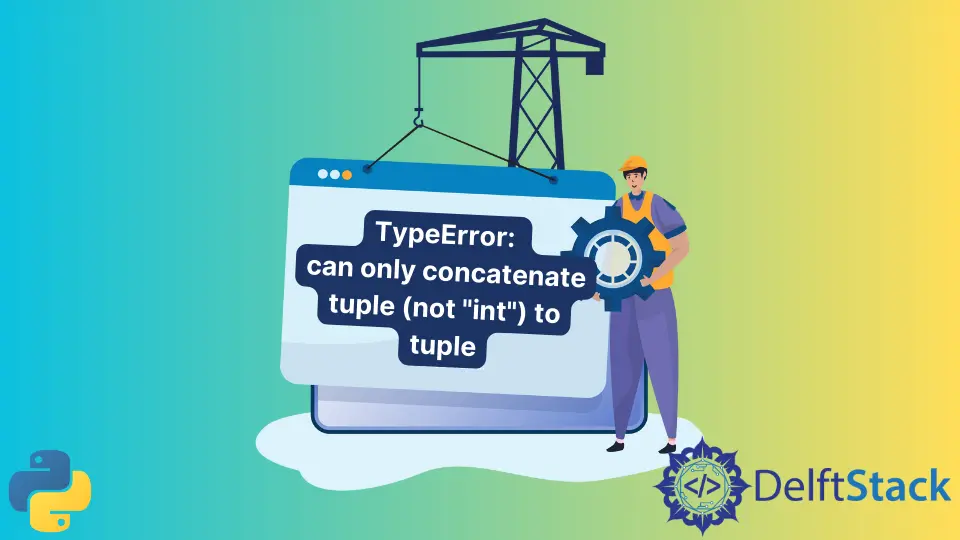
In Python programming language, tuple
is a data structure that can be used to store a collection of objects separated by a comma ,
. The tuple
is immutable, meaning you cannot change its objects.
To create a tuple, you need a name for the tuple and the regular parenthesis ( )
and add an object in it separated by a comma ,
.
Syntax of a tuple:
my_tpl = (1, 2, 3, 4, 5, 6)
print(type(my_tpl)) # print the type of my_tpl
print(my_tpl)
Output:
<class 'tuple'>
(1, 2, 3, 4, 5, 6)
Create a Tuple With a Single Object
We have already understood in the above program about tuple creation, but that was a tuple with multiple objects. The creation of a tuple might be a little different from the others.
Code Example:
my_tpl = 1
print(type(my_tpl))
print(my_tpl)
Output:
<class 'int'>
1
This belongs to the int
class, not tuple
, and the reason is to different int
and tuple
we use a comma ,
after the object of a tuple.
Code Example:
my_tpl = (1,)
print(type(my_tpl))
print(my_tpl)
Output:
<class 'tuple'>
(1,)
We have defined a tuple with a single object in it.
Fix the TypeError: Can Only Concatenate Tuple (Not "Int") To Tuple
in Python
This common error occurs when you try to concatenate the value or values of any data type other than a tuple. Adding an integer to a tuple can cause this error.
Let’s see why this error occurs and how to fix it.
Code Example:
nums_tpl = (1, 2, 3, 4, 5) # Tuple
num_int = 6 # Integer
# Concatinating a tuple and an integer
concatinate = nums_tpl + num_int
print(concatinate)
Output:
TypeError: can only concatenate tuple (not "int") to tuple
Concatenating an integer to a tuple is not allowed in Python, which is why the TypeError
occurs.
To fix the TypeError: can only concatenate tuple (not "int") to tuple
, we can use a tuple instead of an integer because we can concatenate two tuples but not a tuple with any other data type.
Code Example:
nums_tpl = (1, 2, 3, 4, 5) # Tuple
num_int = (6,) # Tuple
# Concatinating two tuples
concatinate = nums_tpl + num_int
print(concatinate)
Output:
(1, 2, 3, 4, 5, 6)
As you can see, the TypeError
is fixed by concatenating two tuples instead of a tuple and an integer.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python