How to Create Turtle Shapes in Python
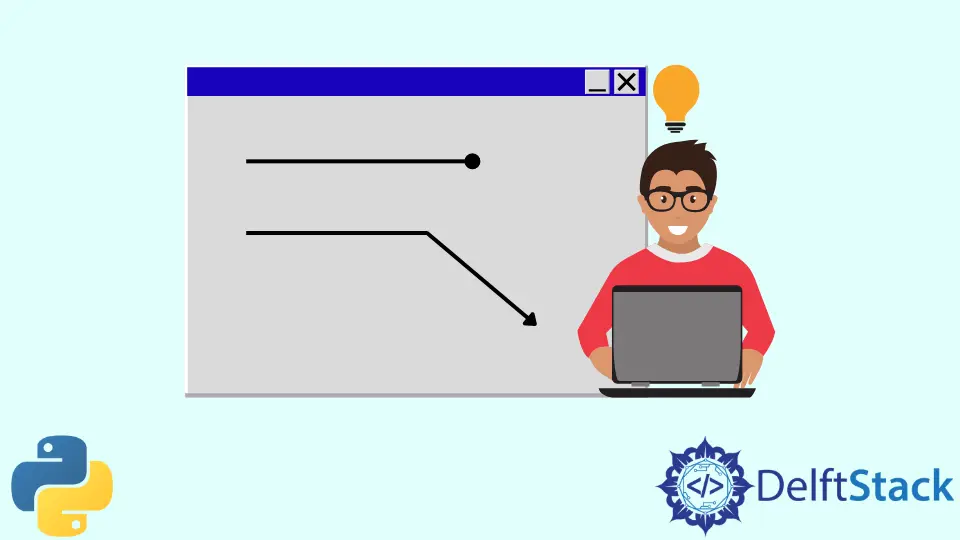
This tutorial explains how to work with the turtle
library and create different shapes in Python. We start by first importing the turtle
library.
Use the turtle.shape()
Function to Create Shapes in Python
Importing turtle
:
import turtle
Turtle
library needs a Python version installed, supporting the Tkinter
library since it uses Tk
for the underlying graphics.
We will work here with the turtle.shape()
function to set the turtle shape to a shape with the given name. The shape name given must exist in the Turtle Screen’s shape library.
We can choose between circle, square, turtle, arrow, triangle and classic.
Let us now start working with the turtle.shape()
function.
turtle.forward(70)
turtle.shape("circle")
turtle.right(60)
turtle.forward(100)
turtle.shape("arrow")
turtle.right(60)
turtle.forward(100)
In the above code, we first create the default shape. Then the circle and arrow shapes, respectively.
Let’s see the above code’s output below.
We have successfully created the turtle shapes using the code above. Hence we can successfully create shapes using the above method.