How to Compare Python Tuples
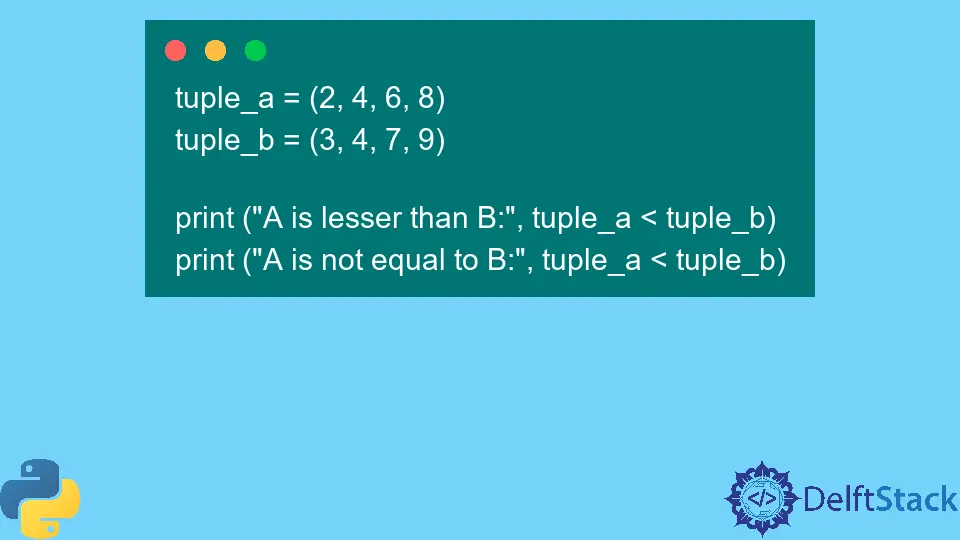
This tutorial will introduce how to compare tuples in Python.
Tuples are compared much like strings and lists. They compare element by element, starting with the first element of the two tuples. First, it checks if the two elements are of the same type. If so, they are then compared by value to identify which is greater, lesser, or equal, depending on the operator.
The comparison is what is called a lexicographical comparison.
Python Tuple Inequality Comparison
For example, determining which tuple is greater will look like this:
tuple_a = (2, 4, 6, 8)
tuple_b = (3, 4, 7, 9)
print("A is greater than B:", tuple_a > tuple_b)
Output:
A is greater than B: False
The output is False
because by comparing the first elements (2 > 3), the result will be false. Comparing the other remaining elements is neglected because there is a conclusive comparison from the first element.
Now, let’s see the other inequality operators’ results, lesser than <
and not equal to !=
, using the same example.
tuple_a = (2, 4, 6, 8)
tuple_b = (3, 4, 7, 9)
print("A is lesser than B:", tuple_a < tuple_b)
print("A is not equal to B:", tuple_a < tuple_b)
Output:
A is lesser than B: True
A is not equal to B: True
Both equate to True
because the comparison of the first elements is already conclusive. 2 is lesser than 3, and they are not equal.
Python Tuple Equality Comparison
In comparing equalities, all elements would need to be compared to be True
. The comparison will stop if there is an inequality.
tuple_a = ("a", "b", "c", "d")
tuple_b = ("a", "b", "c", "d")
print("A is equal to B:", tuple_a == tuple_b)
Output:
A is equal to B: True
Let’s try an example with different types. Declare tuples with a variety of strings, integers, and floats.
tuple_a = ("a", 7, 0.5, "John")
tuple_b = ("a", "c", 0.5, "Jane")
print("A is equal to B:", tuple_a == tuple_b)
Output:
A is equal to B False
Instead of outputting an exception, the output will display a False
value if two elements with different types are compared.
In this example, the first elements are equal, so the comparison moves on to the second elements, which are of integer and string types, respectively. The result will output to False
because they are of different data types.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn