How to Get the Timezones List Using Python
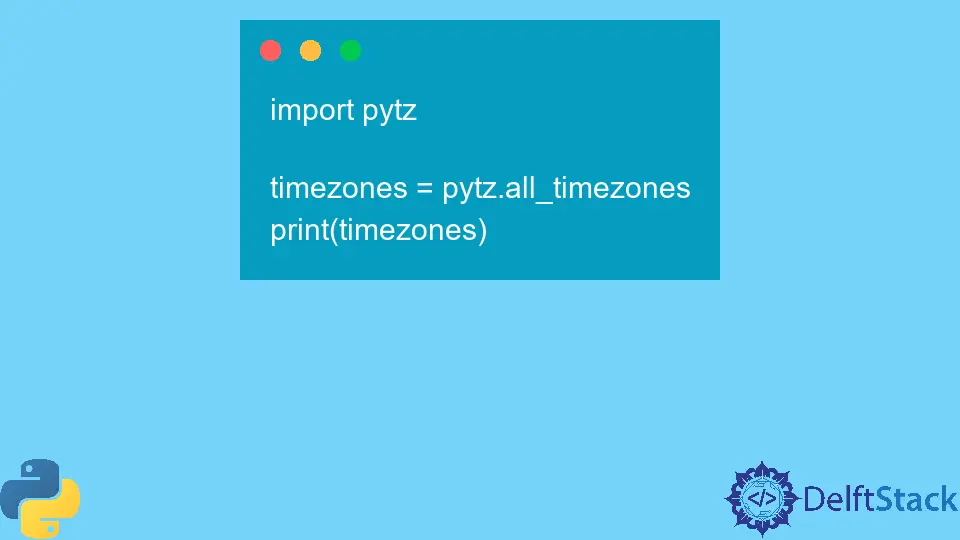
When developing real-world applications, a software developer has to ensure that the application can support users from the home country and other parts of the world. Since most countries have different timezones and many people worldwide keep flying across countries for work and travel purposes, the absolute date and time values can not be stored in the databases.
To counter that, we store UTC or Coordinated Universal Time
or Standard time
values in the databases. They are converted to the actual timezone based on the user’s current location on the fly.
Now, there are many timezones, and rewriting them every time we start a new development project is similar to reinventing the wheel. Hence, developers have created libraries containing all these timezones and utilities to play around with date, time, and timezones.
This article will introduce how to get the timezone list using Python.
Get the Timezones List Using Python
Python has a module, pytz
, containing the Olson tz
database or timezone database. This library allows developers to accurately perform cross timezone calculations such as conversion and daylight saving time. We can use this library to retrieve all the available timezones.
To install this library using the following pip
command.
pip install pytz
Now, let us understand how to use this library to get all the available timezones with the help of the following Python code.
import pytz
timezones = pytz.all_timezones
print(timezones)
Output:
['Africa/Abidjan', 'Africa/Accra', 'Africa/Addis_Ababa', 'Africa/Algiers', 'Africa/Asmara', 'Africa/Asmera', 'Africa/Bamako', 'Africa/Bangui', 'Africa/Banjul', 'Africa/Bissau', ..., 'US/Central', 'US/East-Indiana', 'US/Eastern', 'US/Hawaii', 'US/Indiana-Starke', 'US/Michigan', 'US/Mountain', 'US/Pacific', 'US/Samoa', 'UTC', 'Universal', 'W-SU', 'WET', 'Zulu']
The pytz
library has a variable all_timezones
, which is a list and it contains all the available timezones. This module also has a variable all_timezones_set
, a set of all the available timezones.
The output of the above Python code has a ...
because the number of timezones is too big, and the output has been trimmed for a better understanding. If you wish to see all the available timezones, execute the above Python code.
To learn more about the
pytz
Python library, refer to the official documentation here.