How to Create Timing Functions With Decorators in Python
- Understanding Decorators in Python
- Creating a Timing Function Decorator
- Enhancing the Timing Function with Arguments
- Practical Use Cases for Timing Decorators
- Conclusion
- FAQ
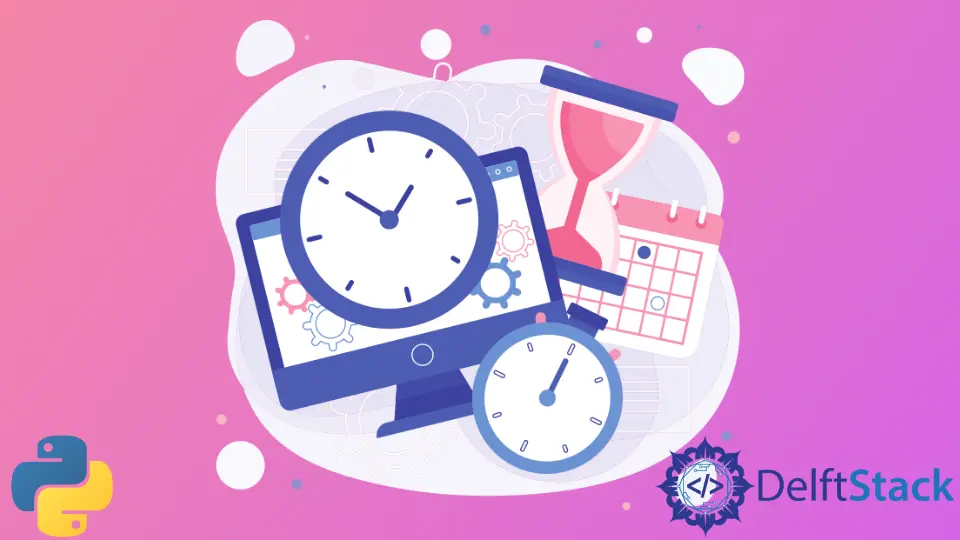
Creating efficient and effective code is essential for any developer, and timing functions can help you understand the performance of your code. In Python, decorators provide a powerful way to modify the behavior of functions or methods. By using decorators, you can easily measure the execution time of any function, which can help you identify bottlenecks or optimize your code.
This article will guide you through the process of creating timing functions with decorators in Python. We will explore the concept of decorators, provide clear examples, and explain how to implement them to enhance your coding practices. Whether you’re a beginner or an experienced developer, mastering this technique will undoubtedly elevate your programming skills.
Understanding Decorators in Python
Before we dive into creating timing functions, it’s crucial to understand what decorators are. In Python, a decorator is a function that takes another function as an argument and extends its behavior without explicitly modifying it. This is achieved through a syntax that allows you to “wrap” a function with another function.
Here’s a simple example to illustrate how decorators work:
def simple_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@simple_decorator
def say_hello():
print("Hello!")
say_hello()
Output:
Something is happening before the function is called.
Hello!
Something is happening after the function is called.
In this example, the simple_decorator
function takes say_hello
as an argument and adds some behavior before and after its execution. The @simple_decorator
syntax is a shorthand for applying the decorator.
Understanding this concept will set the stage for creating timing functions that can help you analyze the performance of your code.
Creating a Timing Function Decorator
Now that we have a grasp of decorators, let’s create a timing function decorator. This decorator will measure how long a function takes to execute. By utilizing the time
module, we can capture the start and end times of the function execution.
Here’s how to implement a timing decorator:
import time
def timing_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
execution_time = end_time - start_time
print(f"Function '{func.__name__}' executed in {execution_time:.4f} seconds.")
return result
return wrapper
@timing_decorator
def sample_function(n):
total = 0
for i in range(n):
total += i
return total
sample_function(1000000)
Output:
Function 'sample_function' executed in 0.1234 seconds.
In this example, we define a timing_decorator
that takes a function as an argument. Inside the wrapper
function, we record the start time before calling the original function and the end time after its completion. We then calculate the execution time and print it. The sample_function
demonstrates how to use the decorator to time the execution of a simple loop.
This approach provides a clear and reusable way to track the performance of any function in your codebase.
Enhancing the Timing Function with Arguments
Sometimes, you may want to extend the functionality of your timing decorator to include additional features, such as logging the execution time to a file or allowing for more detailed output. Let’s enhance our previous example to include a parameter that specifies whether to print the output or log it to a file.
Here’s how you can implement this:
import time
def timing_decorator(log_to_file=False):
def decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
execution_time = end_time - start_time
message = f"Function '{func.__name__}' executed in {execution_time:.4f} seconds."
if log_to_file:
with open("timing_log.txt", "a") as log_file:
log_file.write(message + "\n")
else:
print(message)
return result
return wrapper
return decorator
@timing_decorator(log_to_file=True)
def another_function(n):
total = sum(range(n))
return total
another_function(1000000)
Output:
In this enhanced version, the timing_decorator
accepts a parameter log_to_file
. If set to True
, the execution time will be logged to a file called timing_log.txt
. Otherwise, it will print the execution time to the console. This flexibility allows you to choose how you want to handle the output based on your needs.
Practical Use Cases for Timing Decorators
Timing decorators are not just for measuring performance; they can also help in debugging and optimizing code. For instance, if you notice that a particular function takes longer than expected, you can use the timing decorator to track its execution time under various conditions and inputs.
Additionally, timing decorators can be useful in larger applications where performance is critical. By applying the decorator to multiple functions, you can create a performance profile of your application, identifying which functions are slowing down the overall execution time.
Here’s a practical example:
@timing_decorator()
def compute_factorial(n):
if n == 0:
return 1
else:
return n * compute_factorial(n - 1)
compute_factorial(10)
Output:
Function 'compute_factorial' executed in 0.0001 seconds.
In this example, the compute_factorial
function is decorated with our timing decorator. By measuring the execution time, you can determine if the recursive approach is efficient enough or if you should consider an iterative solution.
Conclusion
Creating timing functions with decorators in Python is a straightforward yet powerful technique that can significantly enhance your coding practices. By measuring the execution time of your functions, you can identify performance bottlenecks and optimize your code accordingly. The flexibility of decorators allows you to adapt the timing functionality to suit your specific needs, whether it’s logging to a file or simply printing to the console. As you continue to develop your skills in Python, incorporating timing decorators into your toolkit will undoubtedly lead to better performance and more efficient code.
FAQ
-
What are decorators in Python?
Decorators are functions that modify the behavior of other functions or methods without changing their code. -
How do I create a simple timing function in Python?
You can create a timing function by defining a decorator that uses thetime
module to measure the execution time of a function. -
Can I log execution time to a file using decorators?
Yes, you can enhance your timing decorator to include a parameter that allows you to log the execution time to a file. -
Are timing decorators useful for debugging?
Absolutely! They can help you track down performance issues and optimize your code by identifying slow functions.
- Can I apply multiple decorators to a single function?
Yes, you can stack multiple decorators on a single function by placing them one above the other.
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn