The Telnetlib Module in Python
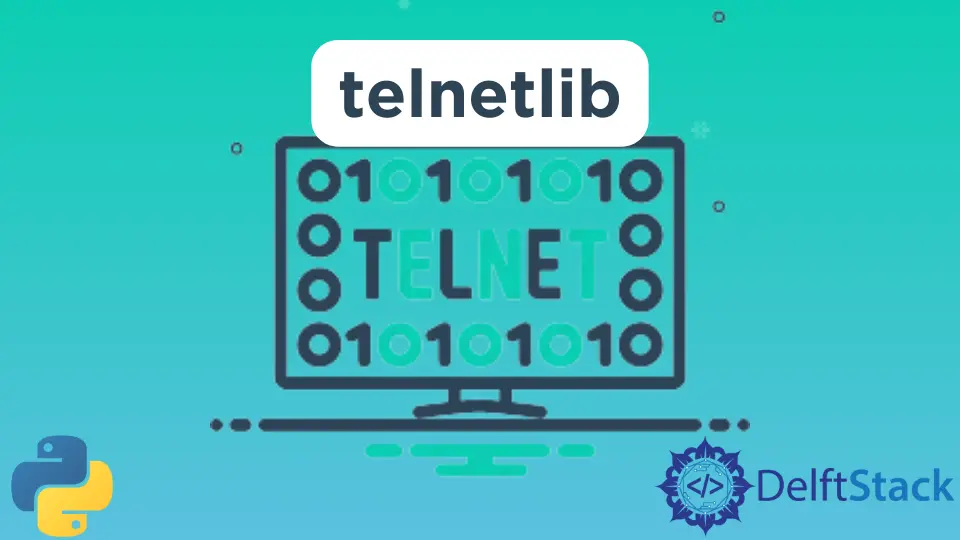
Telnet protocol, developed in 1969 standardized as IETF or Internet Engineering Task Force, is a client-server protocol based on reliable connection-oriented transport.
It is an application protocol used on the LAN or Local Area Network and the Internet. Telnet protocol provides a bidirectional (in both directions) interactive text-oriented communication facility via a virtual terminal (a computer terminal or an electronic hardware device) connection.
In Telnet protocol, the user’s data is scattered over an 8-bit byte-oriented data connection over the TCP or Transmission Control Protocol. The Python programming language has a telnetlib
module that implements the Telnet protocol.
In this article, we will learn about the telnetlib
library.
the telnetlib
Library in Python
The telnetlib
module has a class Telnet
that holds the actual implementation of the Telnet protocol.
This class represents a setup connection to a Telnet server. The Telnet
class constructor accepts two parameters: host
and port
. By default, the host
is None
, and the port
is 0
.
Following is the Telnet
class’s signature.
class telnetlib.Telnet(host=None, port=0)
The Telnet
class has the following methods.
read_until(expected, timeout = None)
- Read until a given byte string,expected
, is found or until thetimeout
amount of seconds have passed by.read_all()
- Read all the data as bytes until EOF or End of File is found. It also blocks until the connection is closed.read_some()
- Read atleast1
byte of data until EOF is encountered.read_very_eager()
- Without blocking the Input-Output or I/O operations, read everything possible.read_eager()
- Read all the readily available data.read_lazy()
- Process and return data already inside the queues.read_very_lazy()
- Return any data that is available in the queue.read_sb_data()
- Return the data which is collected between a SB/SE pair or Suboption Begin/Suboption End.open(host, post = 0)
- Connect to the specified host using the default Telnet protocol port23
. Here,0
points to port23
.msg(msg, *args)
- Print a debugging message when the debug level is more than0
. All the extra arguments are substituted for the message using the standard string formatting operator or%
.set_debuglevel(debuglevel)
- Set the debug level.close()
- Close a connection to the Telnet server.get_socket()
- Return the socket object used internally.fileno()
- Return the file descriptor of the socket object used internally.write(buffer)
- Write a byte string to the socket of the connection.interact()
- It is an interactive function that emulates or matches a very dumb Telnet client.mt_interact()
- A multithreaded interaction function.expect(list, timeout = None)
- Read the data until one regular expression from a list of regular expressions matches.set_option_negotiation_callback(callback)
- Each time a telnet option is read on the input data flow, this callback function is called with the following parameters: callback(telnet socket, command (DO/DONT/WILL/WONT), option). No other operation is performed afterward by thetelnetlib
module.
Example
The following is a straightforward example that tries to depict the usage of the telnetlib
module. Refer to the following Python code.
import getpass
import telnetlib
host = "127.0.0.1"
user = input("Username: ")
password = getpass.getpass()
tn = telnetlib.Telnet(host)
tn.read_until(b"Login: ")
tn.write(user.encode("ascii") + b"\n")
if password:
tn.read_until(b"Password: ")
tn.write(password.encode("ascii") + b"\n")
tn.write(b"ls\n")
tn.write(b"exit\n")
print(tn.read_all().decode("ascii"))
The Python script above will connect to the localhost
or 127.0.0.1
, a loopback address in computer systems.
Next, it will take two inputs: username and password. The getpass
module allows us to take password inputs without visually exposing them safely. Then, the script will connect to the host using the Telnet
class.
It will write the username and password inputs next to the Login:
and Password:
strings. Lastly, it will run the ls
and the exit
command and read all the byte data using the read_all()
method.