How to Fix TabError in Python
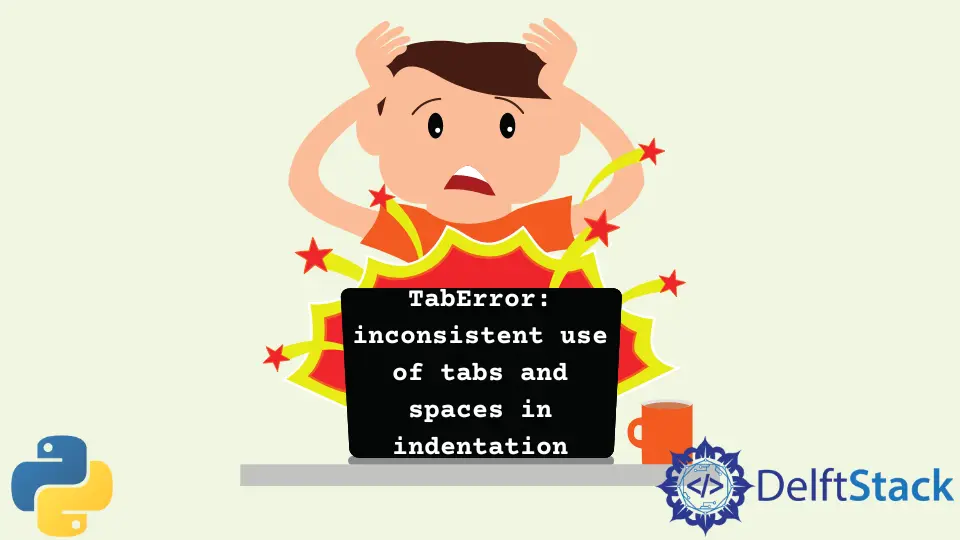
Python is one of the most widely used programming languages. Unlike other programming languages like Java and C++, etc., which uses curly braces for a code block (like a loop block or an if
condition block), it uses indentation to define a block of code.
Indentation Rule in Python
According to the conventions defined, Python uses four spaces or a tab for indentation. A code block starts with a tab indentation, and the next line of code after that block is unindented.
The leading whitespaces determine the indentation level at the beginning of the line. We need to increase the indent level to group the statements for a particular code block.
Similarly, we need to lower the indent level to close the grouping.
Causes of TabError
in Python
Python uses four spaces or a tab for indentation, but if we use both while writing the code, it raises TabError: inconsistent use of tabs and spaces in indentation
. In the following code, we have indented the second and third line using tab and the fourth line using spaces.
Example Code:
# Python 3.x
def check(marks):
if marks > 60:
print("Pass")
print("Congratulations")
check(66)
Output:
#Python 3.x
File "<ipython-input-26-229cb908519e>", line 4
print("Congratulations")
^
TabError: inconsistent use of tabs and spaces in indentation
Fix TabError
in Python
Unfortunately, there is no easy way to fix this error automatically. We have to check each line within a code block.
In our case, we can see the tabs symbol like this ----*
. Whitespaces do not have this symbol. So we can fix the code by consistently using four spaces or tabs.
In our case, we will replace the spaces with tabs to fix the TabError
. Following is the correct code.
Example Code:
# Python 3.x
def check(marks):
if marks > 60:
print("Pass")
print("Congratulations")
check(66)
Output:
#Python 3.x
Pass
Congratulations
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python