How to Fix Python Syntaxerror: Unexpected Character After Line Continuation Character
- Understanding the SyntaxError
- Method 1: Remove Unwanted Characters
- Method 2: Use Parentheses for Line Continuation
- Method 3: Utilize Triple Quotes for Multi-line Strings
- Conclusion
- FAQ
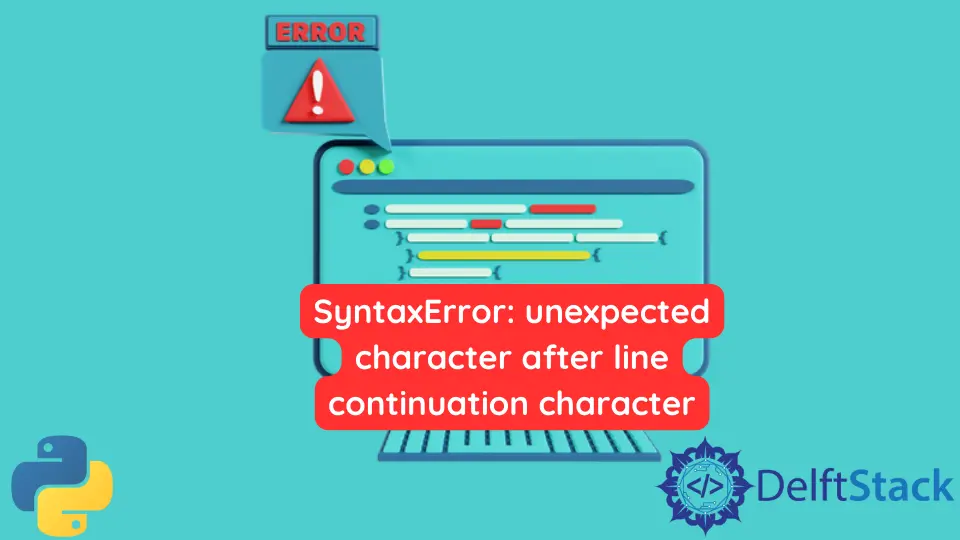
When working with Python, encountering errors can be frustrating, especially when they occur unexpectedly. One such error is the SyntaxError: unexpected character after line continuation character. This error often arises when you try to break a long line of code into multiple lines for better readability, but a small mistake in syntax can lead to this error.
In this article, we will explore what causes this issue and how to fix it effectively. Whether you’re a beginner or an experienced developer, understanding this error will enhance your coding skills and help you write cleaner Python code. So, let’s dive in and learn how to resolve this pesky SyntaxError.
Understanding the SyntaxError
The SyntaxError: unexpected character after line continuation character typically occurs when you use the backslash (\
) to continue a line of code but inadvertently include an invalid character or space after it. Python expects the next line to continue the current statement, but if it finds something unexpected, it raises this error.
For example, consider this incorrect code:
result = 10 + \
20 # Incorrect due to space after backslash
When you run this code, Python will throw a SyntaxError because of the space after the line continuation character.
Output:
SyntaxError: unexpected character after line continuation character
To fix this issue, you need to ensure that there are no characters, including spaces, after the backslash. Let’s look at some methods to resolve this error effectively.
Method 1: Remove Unwanted Characters
The simplest way to fix the SyntaxError is to remove any unwanted characters or spaces after the line continuation character. This method is straightforward and often the first step to troubleshooting the issue.
Consider the following example where a space is present after the backslash:
total = 100 + \
200 # This will raise a SyntaxError
To correct this, simply remove the space after the backslash:
total = 100 + \
200 # Corrected code
Now, when you run the corrected code, it should work without raising an error.
Output:
300
By ensuring there are no spaces or characters after the backslash, you can prevent this SyntaxError from occurring. This solution is simple yet effective, making it a common first step when debugging Python code.
Method 2: Use Parentheses for Line Continuation
Another effective method to avoid the SyntaxError is to use parentheses instead of the backslash for line continuation. This approach not only eliminates the risk of encountering the error but also enhances the readability of your code.
For instance, consider a scenario where you’re adding multiple numbers:
total = (100 +
200 +
300) # Using parentheses for line continuation
This method allows you to break the line naturally without worrying about the backslash. The parentheses indicate that the expression continues on the next line.
Output:
600
Using parentheses not only helps in avoiding the SyntaxError but also makes your code cleaner and easier to read. This technique is particularly useful when dealing with complex expressions or long lists.
Method 3: Utilize Triple Quotes for Multi-line Strings
If your code involves multi-line strings, utilizing triple quotes can be an effective way to avoid the SyntaxError. Triple quotes allow you to create strings that span multiple lines without the need for a line continuation character.
For example, if you want to create a long string that includes line breaks, you can use triple quotes like this:
long_string = """This is a long string
that spans multiple lines
without raising a SyntaxError."""
When you run this code, it will execute without issues, as the triple quotes handle the line breaks internally.
Output:
This is a long string
that spans multiple lines
without raising a SyntaxError.
Using triple quotes is particularly beneficial when you need to include formatted text or documentation within your code. It enhances readability and eliminates the risk of SyntaxErrors related to line continuation.
Conclusion
Encountering the SyntaxError: unexpected character after line continuation character can be a common hurdle for Python developers. However, by understanding the causes and implementing the solutions we’ve discussed, you can easily resolve this issue. Whether it’s removing unwanted characters, using parentheses for line continuation, or utilizing triple quotes for multi-line strings, each method offers a practical approach to writing cleaner and error-free Python code. Embracing these techniques will not only improve your coding efficiency but also enhance the overall quality of your Python projects.
FAQ
-
what causes the SyntaxError: unexpected character after line continuation character?
This error is caused by having an invalid character or space after the line continuation character (\
). -
how can I avoid this error in my Python code?
You can avoid this error by ensuring no characters or spaces follow the backslash or by using parentheses for line continuation. -
is there any benefit to using parentheses instead of backslashes?
Yes, using parentheses improves code readability and eliminates the risk of SyntaxErrors related to line continuation. -
can I use triple quotes to avoid this error?
Yes, triple quotes allow you to create multi-line strings without needing a line continuation character, thus preventing this error. -
what should I do if I still encounter the error after applying these methods?
Review your code for other syntax issues or consult Python documentation for further debugging tips.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python