The super Function in Python
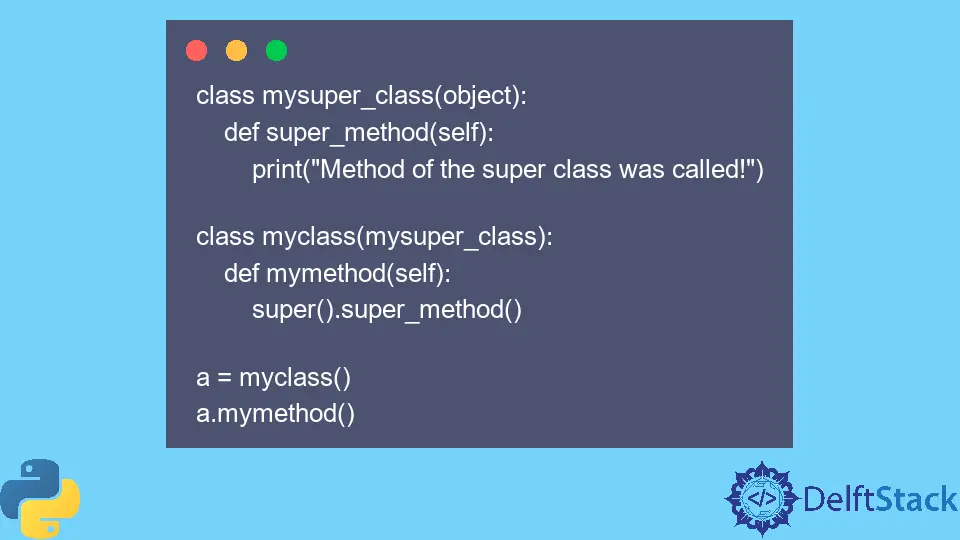
This tutorial will explain the purpose and use of the built-in super()
function in Python. One of the core concepts in Object-Oriented Programming (OOP) is inheritance, in which one class (subclass) can access the properties and methods of the parent class or superclass.
In multiple inheritances, a class can inherit properties and methods from multiple classes, which means the class will have multiple super classes. The super()
function is useful and mainly used in case of multiple inheritances, the details and use of the super()
function with code examples will be discussed in this tutorial.
Use the Built-In Function super()
in Python
The super()
function accesses the overridden inherited methods in a class. The super()
function is used in the child class with multiple inheritance to access the function of the next parent class or superclass. The super()
function determines the next parent class using the Method Resolution Order (MRO). Like if the MRO is C -> D -> B -> A -> object
, for D
, the super()
function will look for the next parent class or superclass method in sequence D -> B -> A -> object
.
If the class is a single inheritance class, in that case, the super()
function is useful to use the methods of the parent classes without using their name explicitly.
The super(type)
function returns a proxy object that calls the methods of the parent or sibling class of the input type
. The syntax of the super()
is different in Python 2 and 3, we can use the super()
function in Python 2 to call inherited method mymethod()
as super(type, self).mymethod(args)
and in Python 3 as super().mymethod(args)
.
Now, let’s look into detailed example codes of using the super()
function to call the inherited methods from the child class in Python.
Example code:
class mysuper_class(object):
def super_method(self):
print("Method of the super class was called!")
class myclass(mysuper_class):
def mymethod(self):
super().super_method()
a = myclass()
a.mymethod()
Output:
Method of the super class was called