How to Sum Dictionary Values in Python
Manav Narula
Feb 02, 2024
-
Sum Values in Python Dictionary With the
for
Loop -
Sum Values in Python Dictionary With the
sum()
Function
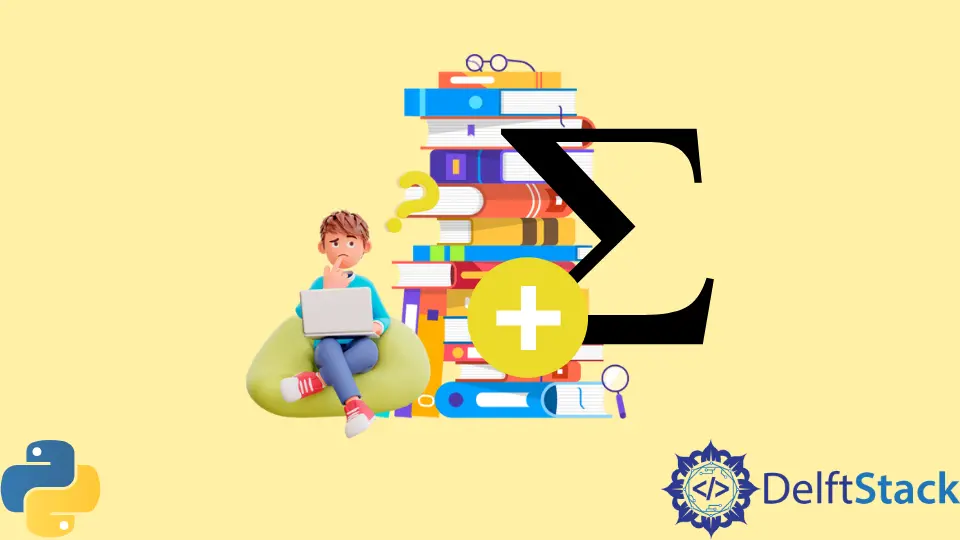
In this tutorial, we will introduce how to sum values in a Python dictionary.
Sum Values in Python Dictionary With the for
Loop
The first method involves the use of the for
loop. We iterate over every value in the dictionary and store the final result in a variable, which is declared outside the loop.
Let’s see the following example.
d1 = {"a": 15, "b": 18, "c": 20}
total = 0
for i in d1.values():
total += i
print(total)
Output:
53
The values()
function returns a list of dictionary values.
Sum Values in Python Dictionary With the sum()
Function
The sum
function is used to return the sum of an interable in Python. We can eliminate the use of the for
loop by using the sum
function. For example,
d1 = {"a": 15, "b": 18, "c": 20}
print(sum(d1.values()))
Output:
53
Author: Manav Narula
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn