How to Fix String Indices Must Be Integers Error in Python
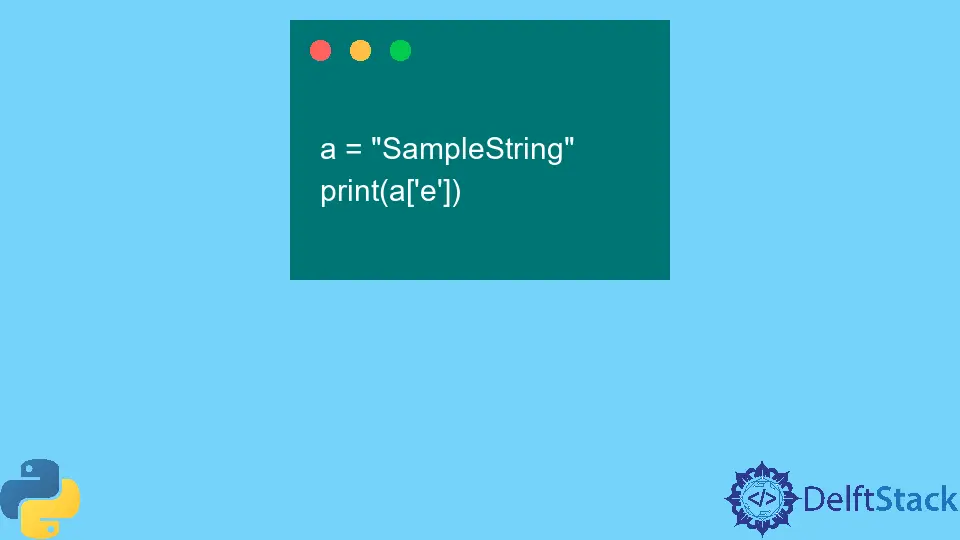
A string is a collection of characters. In Python, it can be thought of as an iterable of characters.
Every character of a string has a specific index. We can easily access characters at different positions using their indexes.
In this article, Python’s string indices must be integers
error. This is a TypeError
.
A TypeError
occurs in Python when an operation with an invalid data type is performed.
Let us now discuss a situation where this error might occur. See the following code.
a = "SampleString"
print(a["e"])
Output:
TypeError: string indices must be integers
You can see that the above code raises the discussed error.
We are trying to access an individual character without its index in the above code. As discussed earlier, we need to provide the character’s index within square brackets to achieve this.
See the code below.
a = "SampleString"
print(a[5])
Output:
e
The above code returns the character e
, since it is at the 6th position.
Note, the first element is stored at the 0th position in Python. The len()
function can be used to return the length of the string.
Another situation where we might encounter this error is while performing a string slicing operation. In string slicing, we extract elements between given positions.
We specify the starting and ending positions within the square brackets. Now, let us see the following example.
a = "SampleString"
print(a[(5, 2)])
Output:
TypeError: string indices must be integers
We pass the indices correctly in the above example, but as a tuple. We need to separate them using a colon :
to overcome this error.
For example:
a = "SampleString"
print(a[2:5])
Output:
mpl
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python