How to Fix STR Object Does Not Support Item Assignment Error in Python
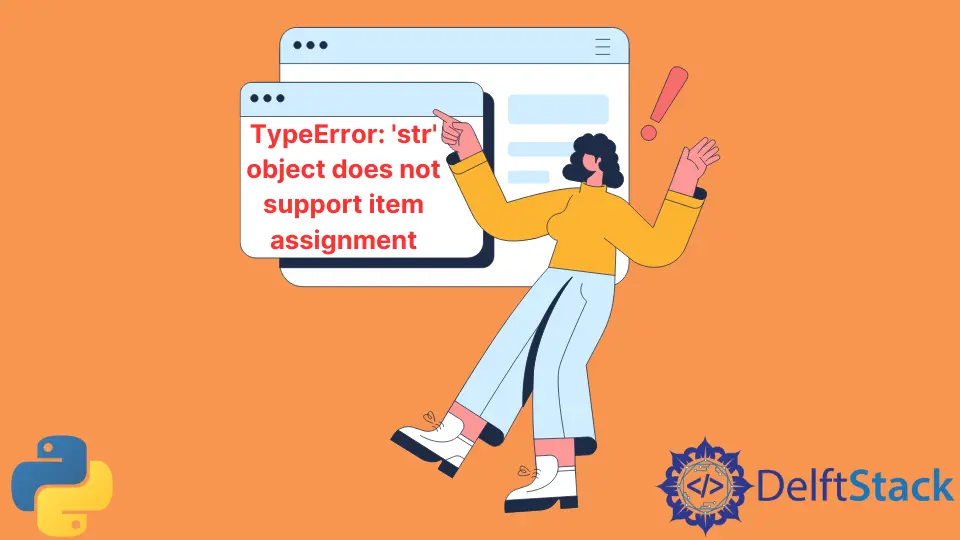
In Python, strings are immutable, so we will get the str object does not support item assignment
error when trying to change the string.
You can not make some changes in the current value of the string. You can either rewrite it completely or convert it into a list first.
This whole guide is all about solving this error. Let’s dive in.
Fix str object does not support item assignment
Error in Python
As the strings are immutable, we can not assign a new value to one of its indexes. Take a look at the following code.
# String Variable
string = "Hello Python"
# printing Fourth index element of the String
print(string[4])
# Trying to Assign value to String
string[4] = "a"
The above code will give o
as output, and later it will give an error once a new value is assigned to its fourth index.
The string works as a single value; although it has indexes, you can not change their value separately. However, if we convert this string into a list first, we can update its value.
# String Variable
string = "Hello Python"
# printing Fourth index element of the String
print(string[4])
# Creating list of String elements
lst = list(string)
print(lst)
# Assigning value to the list
lst[4] = "a"
print(lst)
# use join function to convert list into string
new_String = "".join(lst)
print(new_String)
Output:
o
['H', 'e', 'l', 'l', 'o', ' ', 'P', 'y', 't', 'h', 'o', 'n']
['H', 'e', 'l', 'l', 'a', ' ', 'P', 'y', 't', 'h', 'o', 'n']
Hella Python
The above code will run perfectly.
First, we create a list of string elements. As in the list, all elements are identified by their indexes and are mutable.
We can assign a new value to any of the indexes of the list. Later, we can use the join function to convert the same list into a string and store its value into another string.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python