How to Fix STR Has No Attribute Decode Error in Python
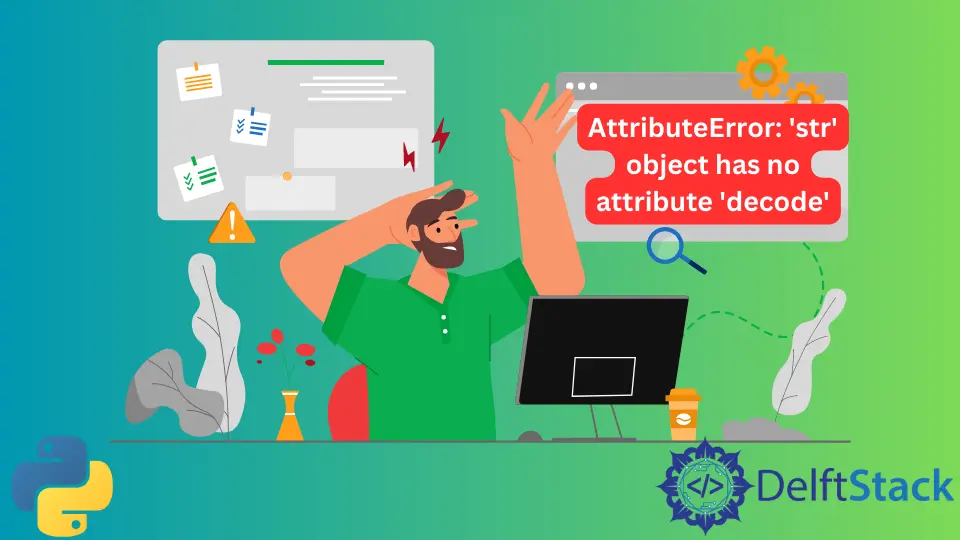
In Python, every entity is considered an object, and every object has some properties or functions associated with it called attributes. The dot operator (.
) is used to invoke these attributes.
In Python 2, the decode
attribute is associated with string objects. This function allows us to transform the encoded data to its original string. We can encode data in different formats and specify the type of encoding used in the decode
function as a parameter.
Sometimes we encounter this 'str' object has no attribute 'decode'
error in Python. It is an AttributeError
, indicating that the decode
attribute is missing from the given string object.
We get this error because, in Python 3, all strings are automatically Unicode objects. Unicode is the format mainly used to encode data. This error is thrown if someone tries to decode a Unicode-encoded object in Python 3.
Below is an example of where we encountered this error.
s = "delftstack"
print(s.decode())
Output:
AttributeError: 'str' object has no attribute 'decode'
The error shows if we decode a string in Python 3. Therefore, we should be careful of the object to decode and ensure it’s not in Unicode format.
We can remove this error by dropping the decode
property from the string object. Another way is by encoding the data first using the encode()
function and then decoding it. This method is redundant but solves the purpose.
For example:
s = "delftstack"
print(s.encode().decode())
Output:
delftstack
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn