SSH Using Python
- Understanding SSH and Its Importance
- Setting Up Your Environment
- Creating an SSH Connection
- Running Multiple Commands
- Handling Errors and Exceptions
- Conclusion
- FAQ
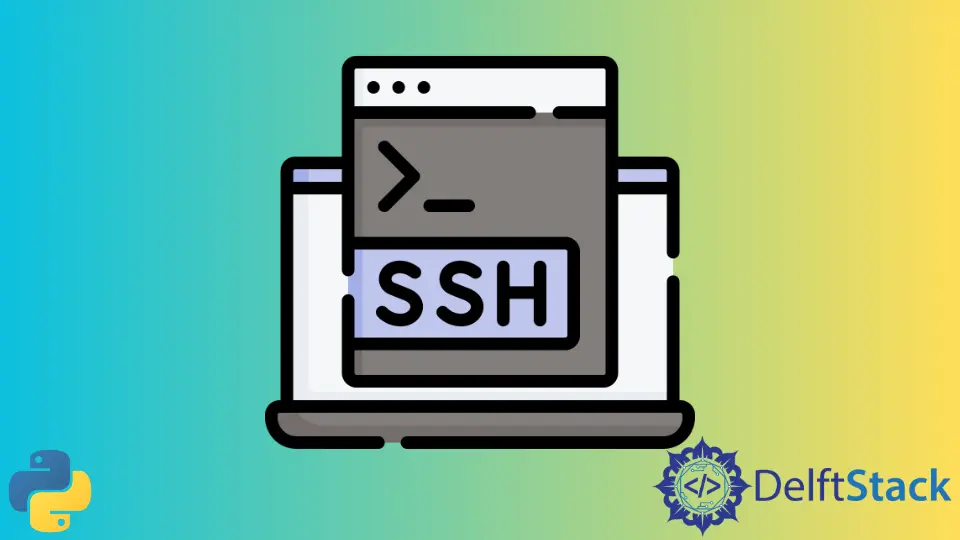
In today’s tech-driven world, managing remote servers efficiently is crucial for developers and system administrators. One of the most powerful tools at your disposal is SSH (Secure Shell), which allows you to securely connect to remote machines. But did you know that you can leverage Python to automate SSH connections and command execution?
This tutorial will guide you through the process of creating SSH connections in Python, enabling you to run commands seamlessly. Whether you’re automating server management tasks or simply wishing to enhance your remote workflow, Python’s libraries make it easier than ever to interact with remote systems. Let’s dive into the world of SSH using Python!
Understanding SSH and Its Importance
SSH, or Secure Shell, is a protocol used to securely access and manage network devices and servers. It encrypts the data exchanged, ensuring that sensitive information remains protected during transmission. For developers and system administrators, SSH is indispensable, allowing for secure remote command execution, file transfers, and even tunneling other protocols.
When combined with Python, SSH becomes a powerful tool for automation. With libraries like Paramiko, you can script interactions with remote servers, making routine tasks simpler and more efficient. This not only saves time but also reduces the risk of human error.
Setting Up Your Environment
Before diving into coding, you’ll need to set up your Python environment. Ensure you have Python installed on your machine. You can download it from the official Python website. Once Python is set up, you’ll need to install the Paramiko library, which provides the functionality to create SSH connections.
To install Paramiko, run the following command in your terminal:
pip install paramiko
This command fetches the library from the Python Package Index (PyPI) and makes it available for use in your projects. With Paramiko installed, you’re ready to start creating SSH connections in Python.
Creating an SSH Connection
Now that your environment is set up, let’s create an SSH connection using Python. Here’s how you can do it:
import paramiko
hostname = 'your_remote_host'
port = 22
username = 'your_username'
password = 'your_password'
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect(hostname, port, username, password)
stdin, stdout, stderr = client.exec_command('ls -l')
print(stdout.read().decode())
client.close()
Output:
total 0
drwxr-xr-x 2 user group 4096 Oct 1 12:00 folder1
drwxr-xr-x 2 user group 4096 Oct 1 12:00 folder2
In this code snippet, we first import the Paramiko library. We define the hostname, port, username, and password required to connect to the remote server. The SSHClient
object is created, and we set a policy to automatically add the server’s host key to known hosts. After connecting to the server, we execute the command ls -l
, which lists the files and directories in the current directory. Finally, we read the output and close the connection.
This method is straightforward and effective for executing basic commands on a remote server.
Running Multiple Commands
Sometimes, you may want to execute multiple commands in a single SSH session. Here’s how you can do that using Python:
import paramiko
hostname = 'your_remote_host'
port = 22
username = 'your_username'
password = 'your_password'
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect(hostname, port, username, password)
commands = ['pwd', 'ls -l', 'whoami']
for command in commands:
stdin, stdout, stderr = client.exec_command(command)
print(f'Output of {command}:\n{stdout.read().decode()}')
client.close()
Output:
Output of pwd:
/home/user
Output of ls -l:
total 0
drwxr-xr-x 2 user group 4096 Oct 1 12:00 folder1
drwxr-xr-x 2 user group 4096 Oct 1 12:00 folder2
Output of whoami:
user
In this example, we define a list of commands to execute. The loop iterates through each command, executing it on the remote server. The output for each command is printed, allowing you to see the results in a structured manner. This method is particularly useful for performing a series of related operations on the remote server without needing to reconnect each time.
Handling Errors and Exceptions
When working with remote connections, it’s essential to handle potential errors gracefully. Here’s how you can implement error handling in your SSH connection code:
import paramiko
hostname = 'your_remote_host'
port = 22
username = 'your_username'
password = 'your_password'
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
try:
client.connect(hostname, port, username, password)
stdin, stdout, stderr = client.exec_command('ls -l')
print(stdout.read().decode())
except paramiko.SSHException as e:
print(f'SSH error: {e}')
except Exception as e:
print(f'Error: {e}')
finally:
client.close()
Output:
total 0
drwxr-xr-x 2 user group 4096 Oct 1 12:00 folder1
drwxr-xr-x 2 user group 4096 Oct 1 12:00 folder2
In this code, we wrap the connection and command execution in a try-except block. If an SSH-related error occurs, it will be caught and printed. This ensures that your program doesn’t crash unexpectedly and provides useful feedback for troubleshooting. The use of the finally block guarantees that the connection is closed, even if an error occurs.
Conclusion
Creating SSH connections using Python is a powerful skill that can significantly enhance your remote server management capabilities. By leveraging the Paramiko library, you can automate various tasks, execute commands, and handle errors effectively. Whether you’re a developer looking to streamline your workflow or a system administrator managing multiple servers, Python provides the tools you need to succeed. With the knowledge gained from this tutorial, you’re now equipped to harness the power of SSH in your Python projects.
FAQ
-
What is SSH?
SSH, or Secure Shell, is a protocol for securely accessing and managing network devices and servers. -
Why use Python for SSH connections?
Python offers libraries like Paramiko that simplify the process of creating SSH connections and automating command execution. -
What is Paramiko?
Paramiko is a Python library that provides an interface for SSH protocol, allowing you to connect to remote servers and execute commands. -
Can I run multiple commands in one SSH session?
Yes, you can execute multiple commands in a single SSH session by iterating through a list of commands. -
How do I handle errors in SSH connections?
You can handle errors by using try-except blocks to catch exceptions related to SSH connections and command execution.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn