How to Sort String Alphabetically in Python
-
Understanding the
sorted()
Function - Sorting a String with Case Sensitivity
- Sorting Words in a Sentence
- Sorting Strings with Custom Criteria
- Conclusion
- FAQ
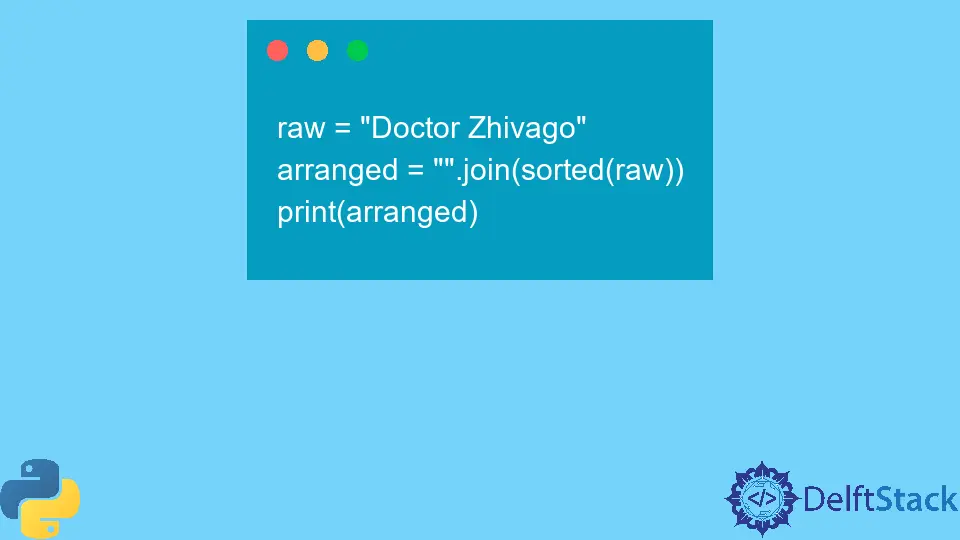
Sorting strings alphabetically is a fundamental task in programming, especially when you need to organize data or present it in a readable format. In Python, the sorted()
function is a powerful and straightforward tool that allows you to accomplish this with ease. Whether you’re working with a list of names, words, or any other string data, mastering this function will enhance your coding skills and improve your ability to manage text data efficiently.
In this article, we’ll explore how to sort strings alphabetically in Python, providing you with clear examples and detailed explanations. By the end, you’ll be equipped with the knowledge to sort strings like a pro!
Understanding the sorted()
Function
The sorted()
function is a built-in Python function that returns a new sorted list from the elements of any iterable, such as strings. When applied to a string, it treats the string as a sequence of characters and sorts them in alphabetical order. Here’s how you can use it:
text = "python programming"
sorted_text = sorted(text)
result = ''.join(sorted_text)
print(result)
Output:
gghimnooprrty
In this example, we start with the string “python programming.” The sorted()
function breaks this string down into its individual characters and sorts them alphabetically. The result is a list of sorted characters, which we then join back together into a single string using join()
. The final output shows the characters in alphabetical order, demonstrating how effectively the sorted()
function can organize string data.
Sorting a String with Case Sensitivity
When sorting strings, it’s essential to understand how case sensitivity affects the order of characters. By default, Python sorts uppercase letters before lowercase ones. This behavior can lead to unexpected results if you aren’t aware of it. Let’s look at an example:
text = "Python programming"
sorted_text = sorted(text)
result = ''.join(sorted_text)
print(result)
Output:
Paaaggimnoprrty
In this case, the uppercase “P” comes first, followed by the lowercase letters. The sorted()
function treats the uppercase “P” as having a lower value than the lowercase letters, which is why it appears at the beginning of the sorted output. If you want to sort the string without considering case sensitivity, you can use the key
parameter of the sorted()
function, like this:
text = "Python programming"
sorted_text = sorted(text, key=str.lower)
result = ''.join(sorted_text)
print(result)
Output:
aaggimmnopprrtyP
By using key=str.lower
, we ensure that all characters are treated the same, regardless of their case. This adjustment gives you a more intuitive alphabetical order, which is often what users expect when sorting strings.
Sorting Words in a Sentence
Sorting isn’t limited to individual characters; you can also sort words within a string. This is particularly useful when you want to organize a sentence or a list of words alphabetically. Here’s how you can achieve this:
sentence = "the quick brown fox jumps over the lazy dog"
words = sentence.split()
sorted_words = sorted(words)
result = ' '.join(sorted_words)
print(result)
Output:
brown dog fox jumps lazy over quick the the
In this example, we first split the sentence into individual words using the split()
method. The sorted()
function is then applied to the list of words, resulting in an alphabetically sorted list. Finally, we join the sorted words back into a single string using join()
. The output shows the words arranged in alphabetical order, demonstrating how you can effectively sort words in a sentence.
Sorting Strings with Custom Criteria
Sometimes, you may want to sort strings based on custom criteria. For instance, you might want to sort words by their length rather than alphabetically. You can achieve this by using a custom function with the key
parameter in the sorted()
function. Here’s an example:
sentence = "the quick brown fox jumps over the lazy dog"
words = sentence.split()
sorted_by_length = sorted(words, key=len)
result = ' '.join(sorted_by_length)
print(result)
Output:
the fox dog over lazy brown quick jumps
In this scenario, we use the len
function as the key for sorting, which sorts the words based on their length. The output shows the words arranged from shortest to longest, highlighting the flexibility of the sorted()
function. You can easily adapt this approach to sort strings by various criteria, making it a versatile tool in your Python programming toolkit.
Conclusion
Sorting strings alphabetically in Python is a straightforward process thanks to the built-in sorted()
function. Whether you’re working with individual characters or entire words, this function offers a flexible and efficient way to organize your data. By understanding how to use the sorted()
function effectively, including its case sensitivity and custom sorting options, you can enhance your programming skills and improve your ability to manage text data. So, the next time you need to sort strings, remember these techniques, and you’ll be sorting like a pro in no time!
FAQ
-
How does the sorted() function work in Python?
The sorted() function takes an iterable and returns a new sorted list of its elements. -
Can I sort strings without considering case sensitivity?
Yes, you can use the key parameter with str.lower to sort strings without case sensitivity. -
How do I sort words in a sentence alphabetically?
You can split the sentence into words, then apply the sorted() function to the list of words. -
Can I sort strings by length instead of alphabetically?
Yes, you can use the key parameter with the len function to sort strings by their length.
- What is the difference between sorted() and sort() in Python?
sorted() returns a new sorted list, while sort() modifies the list in place and does not return a new list.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn