How to Sleep Milliseconds in Python
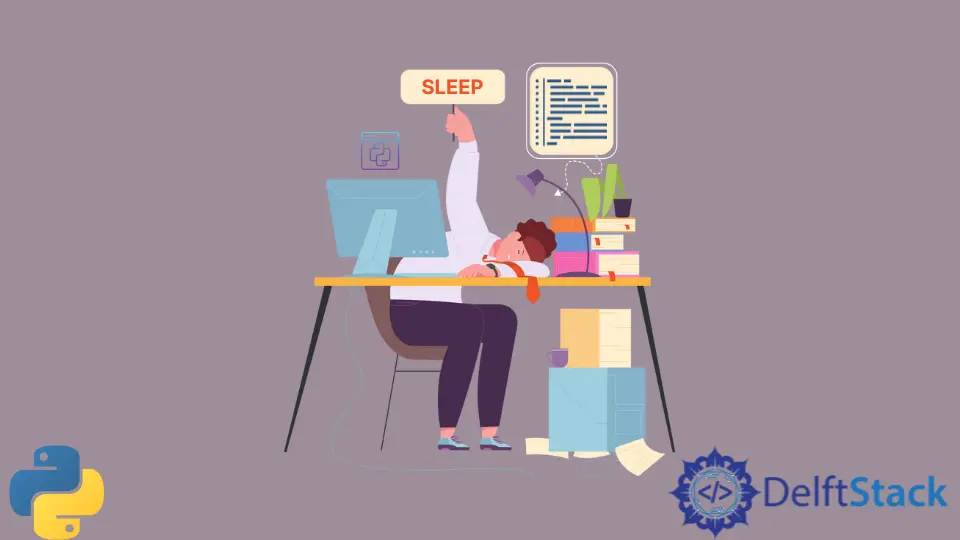
In this tutorial, we will look into various methods to pause or suspend a program’s execution for a given amount of time in Python. Suppose we want to pause the program execution for few seconds to let the user read the instructions about the program’s next step. We need some method to tell the program to go to sleep for a specific number of seconds or milliseconds. We will also discuss a method to make the program call a provided function after a specific time interval without pausing or suspending the program execution
We can use the following methods to pause or suspend the program’s execution for a given amount of time in Python.
Python Sleep Using the time.sleep()
Method
The time.sleep(secs)
method pause or suspends the calling thread’s execution for the number of seconds provided in the secs
argument. Therefore, we need to call the time.sleep()
method to make the program go to sleep for a specific time.
The below example code demonstrates how to use the time.sleep()
method to make the program sleep for the given number of seconds.
import time
time.sleep(1.5)
print("1.5 seconds have passed")
To make the program pause for milliseconds, we will need to divide the input by 1000
, shown in the below example code:
import time
time.sleep(400 / 1000)
print("400 milliseconds have passed")
Python Sleep Using the threading.Timer()
Method
The threading.Timer(interval, function, args, kwargs)
method waits for the time equal to interval
seconds and then calls the function
with arguments args
and keyword arguments kwargs
if provided.
If we want the program to wait for a specific time and then call the function, the threading.Timer()
method will be useful. The below example code demonstrates how to use the threading.Timer()
method to make the program wait for interval
seconds before performing some task.
from threading import Timer
def nextfunction():
print("Next function is called!")
t = Timer(0.5, nextfunction)
t.start()
threading.Timer()
does not pause the program execution, it creates a timer
thread that calls the function after the provided interval
has passed.